Angular 8
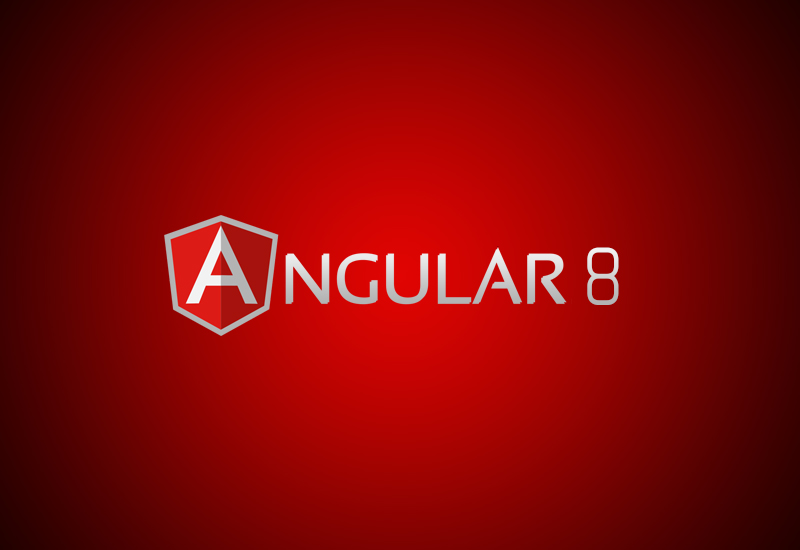
Course Duration
- 1 Total duration - 40+ hours (5 days)
Prerequisites
- 1. Basic HTML and CSS
- 2. Knowledge of JavaScript
- 3. Web development experience is good to have
Learning outcomes:
- 1. Understand Angular key features
- 2. Learn about the Data Flow and usage with Angular
- 3. Implement Bootstrap and CSS to style a Angular app
- 4. Identify the right set of structures and state containers for your angular application
- 5. Manage data by using of angular
- 6. Learn how to handle events and execute angular’s router
- 7. Implement server-side rendering for SEO benefits and to reduce initial load times
- 8. Create, build, and deploy a angular application using the angular CLI
- 9. Develop dynamic Model-driven forms that are easier to unit test
Hands-on Projects :
- 1. Projects on each module covered in this course.
- 2. Angular project: Build an end-to-end WebApp.
Course Overview -Angular:
- ES6:
- 1. Introduction
- 2. Variable and parameters
- a. let - block scope
- b. const
- c. Default parameters
- d. Rest parameters
- e. Spread parameters
- f. Template literals
- 3. Arrays
- a. filter
- b. find
- c. map
- d. reduce
- e. etc
- 4. Arrow functions
- 5. Classes
- a. Declaring class
- b. Inheriting a class
- c. Override
- d. static property
- 6. Modules
- a. Creating a module
- b. Importing a module
- NPM
- 1. Introduction
- 2. Installation
- 3. Installing NPM packages
- 4. How to build and deploy npm packages
- 5. Usages of the npm packages
- a. http-server
- b. typescript
- TypeScripts
- 1. Introduction
- 2. What is TypeScript
- 3. Why and where to use TypeScript
- 4. How TypeScript help in Angular Development
- 5. Why Angular Development is done through TypeScript
- 6. Variables and Functions
- a. Defining Types
- b. String
- c. Int
- d. Bool
- e. Type Inference
- 7. Object types
- 8. Function types
- 9. Arrow Functions
- 10. Classes and Interfaces
- a. Defining Class
- b. Properties
- c. Methods
- d. Extending the Classes
- e. Using Interfaces
- f. Implementing the Interfaces
- 11. Modules
- a. Creating a Module
- b. Using Modules
- c. Module Dependencies
- Angular CLI
- 1. Introduction
- 2. Installing
- 3. How Angular CLI helps in Angular Development
- 4. Create new app
- 5. Create new service
- 6. Create new component
- 7. Create new class
- 8. Create new pipe
- 9. Create new interface
- 10. Run web server etc
- 11. Web Pack- bundling, minification etc
- Angular 2/4/5/6/7/8
- 1. Introduction
- 2. Introduction to MVC
- 3. Why Angular 2/4/5/6/7
- a. create custom Html Elements
- b. Powerful Data Binding
- c. Modular by Design
- d. Built-in Back-End Integration
- 4. Components
- a. Introduction
- b. Template
- c. URL
- d. Class
- e. Properties
- f. Method
- g. Metadata
- h. Decorator
- i. Using As Directive
- j. Modules
- k. Export Module
- l. Import Module
- 5. Data Binding
- a. Interpolation
- b. Property Binding
- c. Two way Binding
- d. Event Binding
- Directives
- 1. Data Binding
- a. Interpolation
- b. Property Binding
- c. Two way Binding
- d. Event Binding
- 2. Structural/Attribute Directives
- a. *ngIf
- b. *ngFor
- 3. Local Variable
- 1. Data Binding
- Pipes
- 1. Introduction
- 2. Built in Pipes
- a. lowercase
- b. uppercase
- c. currency
- d. JSON
- e. date
- f. decimal
- Creative customs pipes
- 1. Create Status Pipe
- 2. Using Status Pipe in Application
- Advanced Components
- Strong Typing and Interfaces
- 1. Specify types to variables
- 2. Create interface and use interface to denote object and arrays
- Component Styles
- 1. Encapsulating styles
- 2. styles
- 3. styleUrls
- HTML controls with binding
- 1. Select Box – two way and *ngFor
- 2. Number – two way
- 3. Button – Event
- Component Lifecycle
- 1. Create
- 2. Render
- 3. Render children
- 4. Process changes
- 5. Destroy
- Component Lifecycle hooks,why and where are used
- 1. OnInit
- 2. OnChanges
- 3. OnDestroy
- Nested Component
- 1. Building Nested Component
- 2. Using Nested Component
- 3. Pass data to Nested Component
- 4. Input binding
- 5. Getting Data Nested Component
- 6. Output binding
- 7. local variable
- 8. local variables
- 9. @ViewChild
- Services and Dependency Injection
- 1. What is service
- 2. Build service
- 3. Register service
- 4. Injecting the service
- Using Built In Services
- 1. HttpClient
- 2. Data Exchange Using HttpClient
- a. Get
- b. Post
- c. Update
- d. Delete
- Observables and Reactive Extensions
- 1. Receiving Data Using Http
- 2. Sending Data using Http
- 3. Handling Errors
- 4. Subscribing to an Observable
- Angular Forms
- 1. Handling User Input
- 2. Binding events to user input.
- 3. Get user input from the $event object
- Template-drive forms
- 1. Template Reference Variable for Control
- 2. Controls status
- a. valid
- b. pristine
- c. dirty
- 3. Template Reference Variable for Form
- 4. Form status
- a. valid
- b. Submitted
- 5. validations
- a. required
- b. pattern
- c. minlength
- 6. Error Messages
- Reactive Forms
- 1. Introduction Dynamic Forms
- 2. Data Model
- 3. FormControl,FormGroup,FormBuilder
- 4. validations
- a. required
- b. pattern
- c. minlength
- 5. Custom Validators
- a. No Space Validators
- 6. Error Messages
- Navigation and Routing
- 1. Routing
- 2. Configuring Routes
- 3. Tying Routes to Actions
- 4. Passing Parameters to a Route
- a. Accessing Route Parameters
- b. routeroutlet
- 5. Activating a Route with Code
- Builtin Directives
- 1. Class
- 2. Style
- 3. Hidden
- Build custom attribute directive
- 1. maxQuantity
- a. ElementRef
- b. Renderer2
- 5. Activating a Route with Code
- 1. maxQuantity
- Build custom structural directive
- 1. Custom ngIF
- Route Guards
- 1. Introduction
- 2. Authentication route guards
- 3. localStorage and sessionStorage
- 4. Authorization route guards
- Modules
- 1. Feature Module
- 2. Root Module
- 3. Routing Module
- 4. Shared Module
- 5. Lazy Loading the Modules
- 6. Eagerly Loading the Modules
- Unit Testing
- 1. Introduction to jasmine and BDD
- 2. Introduction to Test Bed
- 3. Unit testing Class
- Unit testing Angular service
- 1. HttpTestingController
- 2. Mock API Data
- Unit testing Angular component
- 1. nativeElement
- 2. debugElement
- Post Man
- 1. Introduction
- 2. verify
- a. get
- b. post
- c. put
- d. patch
- e. delete
- f. headers in request
- g. body in request
- Chrome Developer Tools
- 1. Elements
- 2. Console
- 3. Network
- 4. Sources
- 5. Application