Microsoft Dot Net
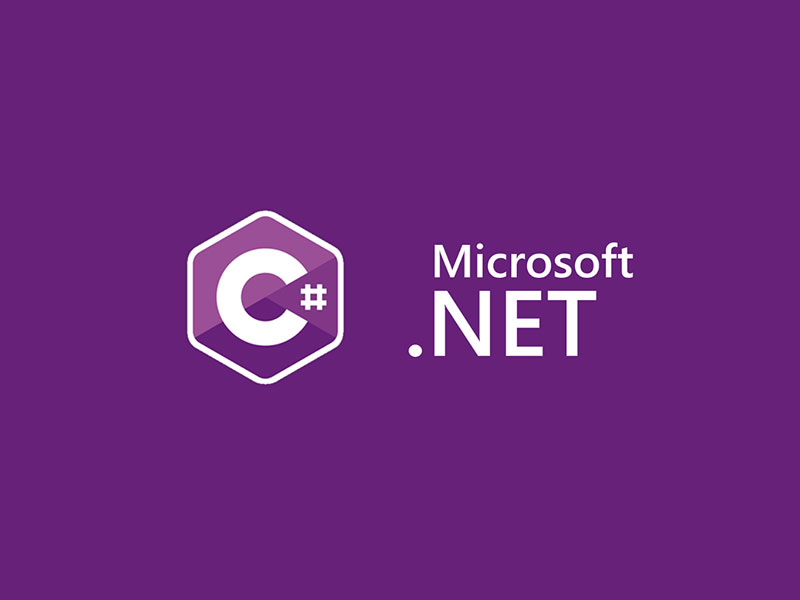
Trainer's Profile :
Microsoft.NET Training is delivered by a real time software professional having more than 10 Years of experience in
Multi National Companies. The trainer has also been onsite and in the United States for 3 years.
Training Approach :
- The Trainer explains the concept from the basics.
- After ensuring that every trainee has well understood the concept, the trainer will move on to explaining how to
apply the same concept to a realtime project. - The trainer will then discuss all the possible interview questions related to the concept in general as well as
relating to a real time project.
MICROSOFT DOTNET CONTENTS
The .NET Framework
- 1. What is the .NET Framework?
- 2. Common Language Runtime
- 3. .NET Framework Class Library
- 4. Assemblies and the Microsoft Intermediate Language (MSIL)
- 5. Versions of the .NET Framework and Visual Studio
Getting Started with Visual Studio
- 1. Visual Studio Overview
- a. Choosing the Development Settings
- b. Resetting the Development Settings
- c. Creating a New Project
- d. Components of the IDE
- 2. Code and Text Editor
- a. Code Snippets
- b. IntelliSense
- c. Refactoring Support
- 3. Debugging
- a. Setting Breakpoints
- b. Stepping through the Code
- c. Watching
- d. Autos and Immediate Windows
- 4. Unit Testing
- a. Creating the Test
- b. Running the Test
- c. Testing with Floating Point Numbers
- d. Adding Additional Test Methods
C# Language Foundations
- 1. Using the C# Compiler (csc.exe)
- 2. Passing Arguments to Main()
- 3. Language Syntax
- a. Keywords
- b. Variables
- c. Scope of Variables
- d. Constants
- e. Comments
- 4. Data Types
- a. Value Types
- b. Reference Types
- c. Enumerations
- d. Implicit Typing
- e. Type Conversion
- 5. Operators
- a. Arithmetic Operators
- b. Logical Operators
- c. Relational Operators
- d. Increment & Decrement Operators
- e. Bit Shift Operators
- f. Assignment Operators
- g. The is and as Operators
- h. Other Operators
- i. Operator Precedence
- 5. Flow Control
- a. if-else Statement
- b. switch Statement
- 6. Looping
- a. for Loop
- b. foreach
- c. while and do-while Loops
- d. Exiting from a Loop
- e. Skipping an Iteration
- 7. Structs
- a. What Are Structs?
- b. Structs Are Value Types
- c. Assigning to a Struct
- d. Constructors and Destructors
- e. Instance Constructors
- f. Static Constructors
- g. Summary of Constructors and Destructors
- h. Field Initializers Are Not Allowed
- i. Structs Are Sealed
- j. Boxing and Unboxing
- k. Structs as Return Values and Parameters
Arrays
- 1. Single Dimensional Arrays
- 2. Accessing Array Elements
- 3. Multidimensional Arrays
- 4. Arrays of Arrays: Jagged Arrays
- 5. Parameter Arrays
- 6. Copying Arrays
Strings and Regular Expressions
- 1. The System.String Class
- a. Escape Characters
- b. String Manipulations
- c. String Formatting
- d. The StringBuilder Class
- 2. Regular Expressions
- a. Searching for a Match
- b. More Complex Pattern Matching
OOPs Concepts
- 1. Classes
- a. Defining a Class
- b. Creating an Instance of a Class (Object Instantiation)
- c. Class Members
- d. Access Modifiers
- e. Function Members
- f. Overloading Methods
- g. Overloading Operators
- h. Using Partial Classes
- i. Static Classes
- 2. System.Object Class
- a. Implementing Equals
- b. ToString() Method
- c. Attributes
- 3. Inheritance
- a. Understanding Inheritance
- b. Implementation Inheritance
- c. Inheritance and Constructors
- d. Calling Base Class Constructors
- e. Virtual Methods (Polymorphism)
- f. Sealed Classes and Methods
- g. Abstract Class
- h. Abstract Methods
- 4. Interfaces
- a. Defining an Interface
- b. Implementing an Interface
- c. Implementing Multiple Interfaces
- d. Interface Inheritance
- e. Overriding Interface Implementations
Collections Interfaces
- 1. Dynamic Arrays Using the ArrayList Class
- 2. Stacks
- 3. Queues
- 4. Dictionary
- 5. Indexers and Iterators
- 6. Implementing IEnumerable and IEnumerator
- 7. Implementing Comparison Using IComparer and IComparable
Delegates and Events
- 1. Delegates
- a. Creating a Delegate
- b. Delegates Chaining (Multicast Delegates)
- c. Implementing Callbacks Using Delegates
- d. Asynchronous Callbacks
- 2. Events
- a. Handling Events
- b. Implementing Events
- c. Difference between Events and Delegates
- d. Passing State Information to an Event Handler
Generics
- 1. Understanding Generics
- 2. Generic Classes
- 3. Using the default Keyword in Generics
- 4. Advantages of Generics
- 5. Using Constraints in a Generic Type
- 6. Generic Interfaces
- 7. Generic Methods
- 8. Generic Operators
- 9. Generic Delegates
- 10. Generics and the .NET Framework Class Library
- 11. System.Collections.ObjectModel
Exception Handling
- 1. Handling Exceptions
- a. Handling Exceptions Using the try-catch Statement
- b. Handling Multiple Exceptions
- c. Throwing Exceptions Using the throw Statement
- d. Using Exception Objects
- e. The finally Statement
- 2. Creating Custom Exceptions
Threading
- 1. Processes, AppDomains, and Object Contexts
- a. Reviewing Traditional Win32 Processes
- b. Interacting with Processes Under the .NET Platform
- c. Understanding .NET Application Domains
- d. Understanding Object Context Boundaries
- 2. Threading
- a. The Need for Multithreading
- b. Starting a Thread
- c. Aborting a Thread
- d. Passing Parameters to Threads
- 3. Thread Synchronization
- a. Using Interlocked Class
- b. Using C# Lock
- c. Monitor Class
- d. Using the BackgroundWorker Control
- e. Testing the Application
Files and Streams
- 1. Working with Files and Directories
- 2. Working with Directories
- 3. Working with Files Using the File and FileInfo Classes
- 4. Creating a FileExplorer
- 5. The Stream Class
- a. BufferedStream
- b. The FileStream Class
- c. MemoryStream
Working with XML
- 1. Introduction to XML
- a. Markup
- b. The Document Type Declaration (DTD)
- c. Encoding Declaration
- d. Writing XML Code using Code Editor
- e. XML Syntax
- 2. XML Reading & Writing
- a. Text Writer Fundamentals
- b. Text Reader Fundamentals
- c. XMLDocument
- 3. Serialization
- a. Binary Serialization
- b. XML Serialization
Assemblies and Versioning
- 1. Assemblies
- 2. Structure of an Assembly
- 3. Examining the Content of an Assembly
- 4. Single and Multi-File Assemblies
- 5. Understanding Namespaces and Assemblies
- 6. Private versus Shared Assemblies
- 7. Creating a Shared Assembly
- 8. The Global Assembly Cache
- 9. Putting the Shared Assembly into GAC
- 10. Making the Shared Assembly Visible in Visual Studio
- 11. Using the Shared Assembly
Type Reflection, Late Binding, and Attribute-Based
- 1. Programming
- 2. The Necessity of Type Metadata
- 3. Understanding Reflection
- 4. Understanding Late Binding
- 5. Building a Custom Metadata Viewer
- 6. Dynamically Loading Assemblies
- 7. Reflecting on Shared Assemblies
- 8. Understanding Attributed
- 9. Assembly-Level (and Module-Level) Attributes
- 10. Reflecting on Attributes Using Early Binding
- 11. Reflecting on Attributes Using Late Binding
- 12. Putting Reflection, Late Binding, and Custom Attributes in Perspective
ADO.NET
- 1. ADO .NET Introduction
- a. ADO vs. ADO .NET
- b. ADO .NET Architecture
- 2. Connecting to Data Sources
- a. Choosing a .Net Data Provider
- b. Defining a Connection
- c. Managing a Connection
- d. Handling Connection Exceptions
- e. Connection Pooling
- 3. Performing Connected Database Operations
- a. Working in a Connected Environment
- b. Building Command Objects
- c. Executing the Commands
- d. Data Reader Object
- 4. Using Transactions
- 5. Perfoming Dis-Connected Database Operations
- a. Configuring a DataAdapter to Retrieve Information
- b. Populating a DataSet Using a DataAdapter
- c. Modifying Data in a DataTable
- d. Persisting Changes to a Data Source
- e. Working in a Disconnected Environment
- f. Building Datasets and DataTables
- g. Binding and Saving a Dataset
- h. Defining Data Relationships
- 6. Reading and Writing XML with ADO.NET
- a. Creating XSD Schemas
- b. Loading Schemas and Data into DataSets
- c. Writing XML from a DataSet
ASP.NET
- 1. ASP.NET Introduction
- a. The Evolution of Web Development
- b. HTML and HTML Forms
- c. Server-Side Programming
- d. Client-Side Programming
- e. Facts about ASP .NET
- f. ASP .NET different versions
- 2. Developing ASP.NET Applications
- a. Developing ASP.NET Applications
- i. Creating a New Web Application
- ii. Websites and Web Projects
- iii. The Hidden Solution Files
- iv. The Solution Explorer
- v. Adding Web Forms
- vi. Migrating a Website from a Previous Version of Visual Studio
- b. Designing a Web Page
- i. Adding Web Controls
- ii. The Properties Window
- c. The Anatomy of a Web Form
- i. The Web Form Markup
- ii. The Page Directive
- iii. The Doctype
- d. Writing Code
- i. The Code-Behind Class
- ii. Adding Event Handlers
- iii. IntelliSense and Outlining
- e. Visual Studio Debugging
- i. The Visual Studio Web Server
- ii. Single-Step Debugging
- iii. Variable Watches
- a. Developing ASP.NET Applications
Creating N-Tier Applications
- 1. Multi-Tier Architectures
- 2. Creating an N-Tier ASP.NET Application
- 3. The Data Tier
- 4. The Middle Tier
- 5. The Presentation Tier
- 6. Managing Concurrency
Web Form Fundamentals
- 1. The Anatomy of an ASP.NET Application
- a. ASP.NET File Types
- b. ASP.NET Application Directories
- 2. Introducing Server Controls
- a. HTML Server Controls
- b. Converting an HTML Page to an ASP.NET Page
- 3. A Deeper Look at HTML Control Classes
- a. HTML Control Events
- b. Advanced Events with the HtmlInputImage Control
- c. The HtmlControl Base Class
- d. The HtmlContainerControl Class
- e. The HtmlInputControl Class
- 4. The Page Class
- a. Sending the User to a New Page
- b. HTML Encoding
- 5. Application Events
- a. The Global.asax File
- b. Additional Application Events
- 6. ASP.NET Configuration
- a. The web.config File
- b. Nested Configuration
- c. Storing Custom Settings in the web.config File
- d. The Website Administration Tool (WAT)
Web Controls
- 1. Stepping Up Web Controls
- a. Basic Web Control Classes
- b. The Web Control Tags
- 2. Web Control Classes
- a. The WebControl Base Class
- b. Units
- c. Enumerations
- d. Colors
- e. Fonts
- f. Focus
- g. The Default Button
- 3. List Controls
- a. Multiple-Select List Controls
- b. The BulletedList Control
- 4. Table Controls
- 5. Web Control Events and AutoPostBack
- a. How Postback Events Work
- b. The Page Life Cycle
- 6. A Simple Web Page
- a. Improving the Greeting Card Generator
- b. Generating the Cards Automatically
Validation
- 1. Understanding Validation
- a. The Validator Controls
- b. Server-Side Validation
- c. Client-Side Validation
- 2. The Validation Controls
- a. A Simple Validation Example
- b. Other Display Options
- c. Manual Validation
- d. Validating with Regular Expressions
- e. A Validated Customer Form
- f. Validation Groups
The Data Controls
- 1. The GridView
- a. Automatically Generating Columns
- b. Defining Columns
- 2. Formatting the GridView
- a. Formatting Fields
- b. Using Styles
- c. Formatting-Specific Values
- 3. Selecting a GridView Row
- a. Adding a Select Button
- b. Editing with the GridView
- 4. Sorting and Paging the GridView
- a. Sorting
- b. Paging
- 5. Using GridView Templates
- a. Using Multiple Templates
- b. Editing Templates in Visual Studio
- c. Handling Events in a Template
- d. Editing with a Template
- 6. The DetailsView and FormView
- a. The DetailsView
- b. The FormView
Rich Controls
- 1. The Calendar
- a. Formatting the Calendar
- b. Restricting Dates
- 2. The AdRotator
- a. The Advertisement File
- b. The AdRotator Class
- 3. Pages with Multiple Views
- a. The MultiView Control
- b. The Wizard Control
Files and Streams
- 1. Files and Web Applications
- 2. File System Information
- a. The Path Class
- b. A Sample File Browser
- 3. Reading and Writing with Streams
- a. Text Files
- b. Binary Files
- c. Shortcuts for Reading and Writing Files
- d. A Simple Guest Book
- 4. Allowing File Uploads
- a. The FileUpload Control
State Management
- 1. The Problem of State
- 2. View State
- a. The ViewState Collection
- b. A View State Example
- c. Making View State Secure
- d. Retaining Member Variables
- e. Storing Custom Objects
- 3. Transferring Information between Pages
- a. Cross-Page Posting
- b. The Query String
- 4. Cookies
- a. A Cookie Example
- 5. Session State
- a. Session Tracking
- b. Using Session State
- c. A Session State Example
- 6. Session State Configuration
- a. Cookieless
- b. Timeout
- c. Mode
- 7. Application State
- 8. An Overview of State Management Choices
Error Handling, Logging, and Tracing
- 1. Common Errors
- 2. Exception Handling
- a. The Exception Class
- b. The Exception Chain
- 3. Handling Exceptions
- a. Catching Specific Exceptions
- b. Nested Exception Handlers
- c. Exception Handling in Action
- d. Mastering Exceptions
- 4. Throwing Your Own Exceptions
- 5. Logging Exceptions
- a. Viewing the Windows Event Logs
- b. Writing to the Event Log
- c. Custom Logs
- d. A Custom Logging Class
- e. Retrieving Log Information
- 6. Error Pages
- a. Error Modes
- b. Custom Error Pages
- 7. Page Tracing
- a. Enabling Tracing
- b. Tracing Information
- c. Writing Trace Information
- d. Application-Level Tracing
Deploying ASP.NET Applications
- 1. ASP.NET Applications and the Web Server
- a. How Web Servers Work
- b. The Virtual Directory
- c. Web Application URLs
- d. Web Farms
- 2. Internet Information Services (IIS)
- a. The Many Faces of IIS
- b. Installing IIS 5 (in Windows XP)
- c. Installing IIS 7 (in Windows Vista)
- d. Registering the ASP.NET File Mappings
- e. Verifying That ASP.NET Is Correctly Installed
- 3. Managing Websites with IIS Manager
- a. Creating a Virtual Directory
- b. Configuring a Virtual Directory
- 4. Deploying a Simple Site
- a. Web Applications and Components
- b. Other Configuration Steps
- c. Code Compilation
- d. The ASP.NET Account
- 5. Deploying with Visual Studio
- a. Creating a Virtual Directory for a New Project
- b. Copying a Website
- c. Publishing a Website
User Controls and Graphics
- 1. User Controls
- a. Creating a Simple User Control
- b. Independent User Controls
- c. Integrated User Controls
- d. User Control Events
- e. Passing Information with Events
- 2. Dynamic Graphics
- a. Basic Drawing
- b. Drawing a Custom Image
- c. Placing Custom Images Inside Web Pages
- d. Image Format and Quality
Caching
- 1. Understanding Caching
- a. When to Use Caching
- b. Caching in ASP.NET
- 2. Output Caching
- a. Caching on the Client Side
- b. Caching and the Query String
- c. Caching with Specific Query String Parameters
- d. A Multiple Caching Example
- e. Custom Caching Control
- f. Fragment Caching
- g. Cache Profiles
- 3. Data Caching
- a. Adding Items to the Cache
- b. A Simple Cache Test
- c. Caching to Provide Multiple Views
Styles, Themes, and Master Pages
- 1. Styles
- a. Style Types
- b. Creating a Basic Inline Style
- c. Creating a Style Sheet
- d. Applying Style Sheet Rules
- 2. Themes
- a. How Themes Work
- b. Applying a Simple Theme
- c. Handling Theme Conflicts
- d. Creating Multiple Skins for the Same Control
- e. More Advanced Skins
- 3. Master Page Basics
- a. A Simple Master Page and Content Page
- b. How Master Pages and Content Pages Are Connected
- c. A Master Page with Multiple Content Regions
- d. Default Content
- e. Master Pages and Relative Paths
- 4. Advanced Master Pages
- a. Table-Based Layouts
- b. Code in a Master Page
- c. Interacting with a Master Page Programmatically
Website Navigation
- 1. Site Maps
- a. Defining a Site Map
- b. Seeing a Simple Site Map in Action
- c. Binding an Ordinary Page to a Site Map
- d. Binding a Master Page to a Site Map
- e. Binding Portions of a Site Map
- f. The SiteMap Class
- g. Mapping URLs
- 2. The SiteMapPath Control
- a. Customizing the SiteMapPath
- b. Using SiteMapPath Styles and Templates
- c. Adding Custom Site Map Information
- 3. The TreeView Control
- a. TreeView Properties
- b. TreeView Styles
- 4. The Menu Control
- a. Menu Styles
- b. Menu Templates
Creating and Using Web Services
- 1. Understanding the Web Service Model
- 2. Creating an ASP.NET Web Service
- 3. WSDL and Web Service Clients
- 4. Creating & Consuming Web Services with Visual Studio .NET
Security Fundamentals
- 1. Determining Security Requirements
- 2. The ASP.NET Security Model
- a. The Visual Studio Web Server
- b. Authentication and Authorization
- 3. Forms Authentication
- a. Web.config Settings
- b. Authorization Rules
- c. The WAT
- d. The Login Page
- 4. Windows Authentication
- a. Web.config Settings
- b. IIS Settings
- c. A Windows Authentication Test
- 5. Impersonation
- a. Understanding Impersonation
- b. Programmatic Impersonation
- 6. Confidentiality with SSL
- a. Creating a Certificate Request
- b. Secure Sockets Layer
Membership
- 1. The Membership Data Store
- a. Membership with SQL Server
- b. Using the Full Version of SQL Server
- c. Configuring the Membership Provider
- d. Creating Users with the WAT
- e. The Membership and Membership User Classes
- f. Authentication with Membership
- g. Disabled Accounts
- 2. The Security Controls
- a. The Login Control
- b. The CreateUserWizard Control
- c. The PasswordRecovery Control
- 3. Role-Based Security
- a. Creating and Assigning Roles
- b. Restricting Access Based on Roles
- c. The LoginView Control
Profiles
- 1. Understanding Profiles
- a. Profile Performance
- b. How Profiles Store Data
- 2. Using the SqlProfileProvider
- a. Enabling Authentication
- b. Using the Full Version of SQL Server
- c. The Profile Databases
- d. Defining Profile Properties
- e. Using Profile Properties
- f. Profile Serialization
- g. Profile Groups
- h. Profiles and Custom Data Types
- i. The Profile API
- j. Anonymous Profiles
ASP.NET AJAX
- 1. Understanding Ajax
- a. Ajax: The Good
- b. Ajax: The Bad
- c. The ASP.NET AJAX Toolkit.
- d. The ScriptManager
- 2. Partial Refreshes
- a. A Simple UpdatePanel Test
- b. Handling Errors
- c. Conditional Updates
- d. Triggers
- 3. Progress Notification
- a. Showing a Simulated Progress Bar
- b. Cancellation
- c. Timed Refreshes
- 4. The ASP.NET AJAX Control Toolkit
- a. Installing the ASP.NET AJAX Control Toolkit
.NET Version 4.0
- 1. Implicitly Typed Local Variables and Arrays
- 2. Object Initializers
- 3. Collection Initializers
- 4. Extension Methods
- 5. Anonymous Types
- 6. Lambda Expressions
- 7. Query Keywords
- 8. Auto-Implemented Properties
- 9. Language-Integrated Query (LINQ)
- 10. dynamic data type
- 11. Named Parameters
Windows Communication Foundation
- 1. WCF Essentials
- a. WCF Overview
- b. SOA Overview
- c. WCF architecture
- d. Essential WCF concepts:
- e. Addresses
- f. Contracts
- g. Bindings
- h. Endpoints
- i. Hosting
- j. Clients
- 2. Contracts
- a. Designing and working with service contracts
- b. Contract overloading and inheritance
- c. Data Contracts
- d. Serialization
- e. Attributes
- f. Versioning
- g. Collections & Generics
- 3. Instance Management & Operation
- a. Behaviors
- b. Per-Call Services
- c. Per-Session Services
- d. Singleton Service
- e. Demarcating Operations
- f. Instance Deactivation
- g. Throttling
- h. Operations
- i. Request-Reply
- ii. One-Way
- iii. Callback
- iv. Events
- i. Streaming
- 4. Faults
- a. Errors and exceptions
- b. Fault Contracts
- c. Error handling Extensions
- 5. Transactions
- a. Transaction Propagation
- b. Protocols and Managers
- c. The Transaction Class
- d. Declarative Programming
- e. Explicit Transaction Programming
- f. With Instance management
- g. Callbacks
- 6. Security
- a. Authentication & Authorization
- b. Transfer Security
- c. Scenario-Driven Approach
- 7. Concurrency Management
- a. Service Concurrency Mode
- b. Instance Management and Concurrency
- c. Deadlocked Avoidance
- d. Synchronization Context
- e. Callbacks
Project
• A project using .NET programming has to be developed by participant