Best dot net training institute in Marathahalli Bangalore
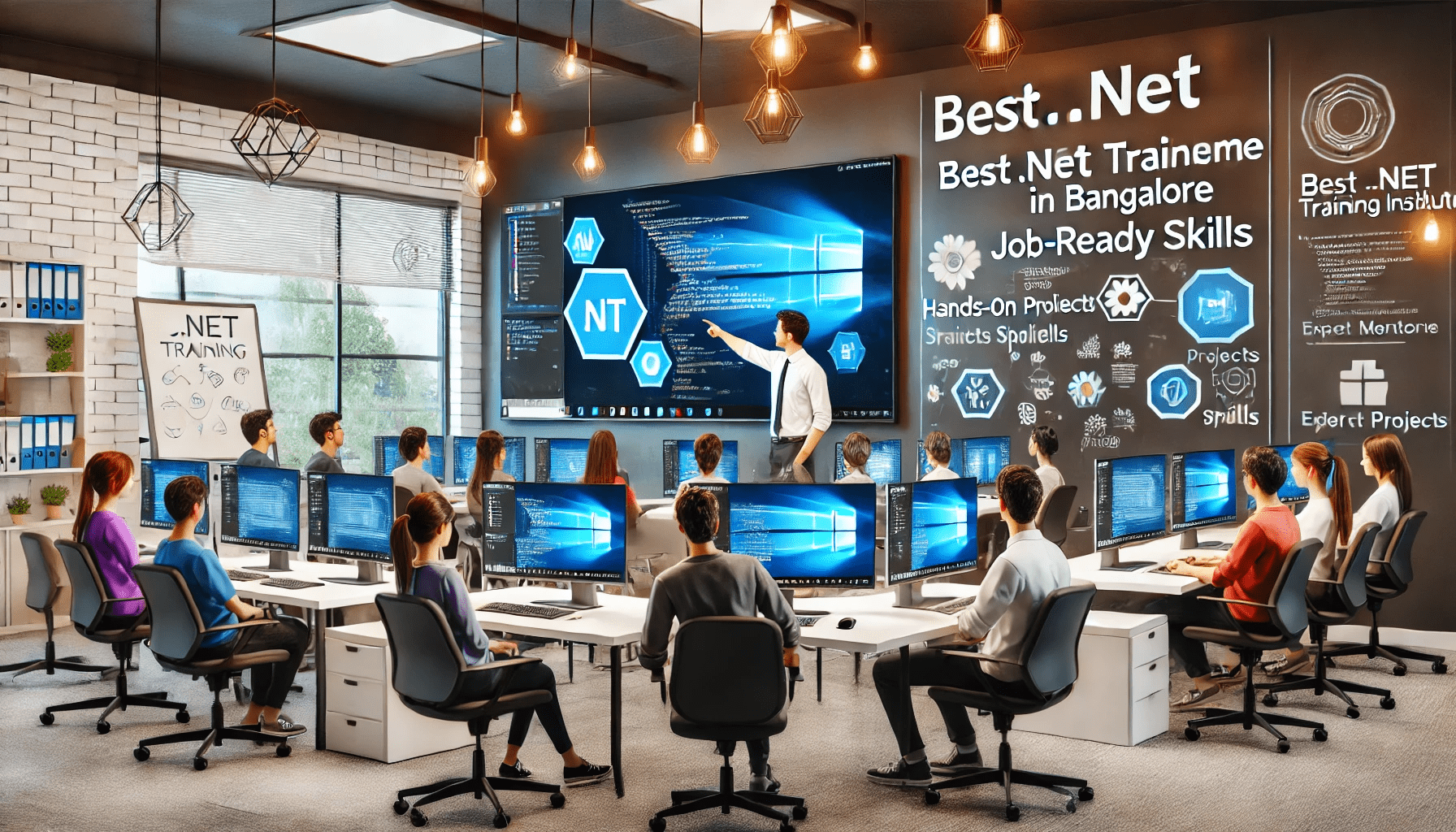
Commonly asked Dot Net Interview Questions and Answers for freshers and experienced.
PragimTech is the best .NET training institute in Marathahalli Bangalore. All these questions were asked in the previous interviews our trainees attended.
Basic C#
What is C#?
C# is a modern, object-oriented, and type-safe programming language developed by Microsoft as part of the .NET framework. It is widely used for developing web, desktop, and mobile applications.
What are the key features of C#?
- Object-Oriented
- Type-Safe
- Platform-Independent with .NET Core
- Rich Library Support
- Automatic Memory Management (Garbage Collection)
- LINQ (Language-Integrated Query)
What is the difference between value type and reference type in C#?
Value Type: Stores the actual data and resides in the stack. Examples: int, float, char.
Reference Type: Stores the reference to the data (address) and resides in the heap. Examples: object, string, arrays.
What are nullable types in C#?
Nullable types allow value types to accept null. Declared using ?. For example, int? num = null;.
What is the var keyword in C#?
var is an implicitly typed variable, meaning the type is inferred at compile-time. Example: var num = 5; (implicitly an integer).
What is the difference between const and readonly in C#?
const: Compile-time constant; value must be assigned when declared.
readonly: Runtime constant; value can be assigned in the constructor.
OOP Concepts
What are the four principles of OOP?
Encapsulation: Binding data and methods together; using access modifiers for control.
Abstraction: Hiding implementation details and exposing only essential features.
Inheritance: Acquiring properties and behaviors from a parent class.
Polymorphism: Same interface, different implementations (overloading and overriding).
Explain encapsulation with an example
class BankAccount { private decimal balance; // Private field (hidden) public void Deposit(decimal amount) { // Public method to add money if (amount > 0) { balance += amount; Console.WriteLine($"Deposited: {amount}"); } else { Console.WriteLine("Deposit amount must be positive!"); } } public decimal GetBalance() { // Public method to view balance return balance; } } class Program { static void Main() { BankAccount account = new BankAccount(); account.Deposit(100); // Depositing money Console.WriteLine($"Balance: {account.GetBalance()}"); // Viewing balance } }
- The
balance
field is private and cannot be accessed directly. - Access to
balance
is controlled through:Deposit(decimal amount)
: Adds money only if the amount is valid.GetBalance()
: Safely retrieves the current balance.
- This ensures the balance is updated and retrieved in a controlled and secure manner, just like in a real bank system.
Encapsulation in this example is the practice of hiding the balance
field (private) to protect it from unauthorized access and providing public methods (Deposit
and GetBalance)
to control and manage how the balance is accessed and updated. It ensures data security and controlled interaction.
Explain abstraction with a real world example
Abstraction focuses on showing only the essential details of an object while hiding the implementation details. It allows you to work with high-level concepts and avoids exposing how things work internally.
Simple Example: Online Payment
Imagine an OnlinePayment system where the user only cares about paying, not how the payment processing works behind the scenes.
abstract class Payment { // Abstract class defining essential behavior public abstract void MakePayment(decimal amount); // Abstract method } class CreditCardPayment : Payment { public override void MakePayment(decimal amount) { // Implementation for Credit Card Console.WriteLine($"Processing credit card payment of {amount}"); } } class PayPalPayment : Payment { public override void MakePayment(decimal amount) { // Implementation for PayPal Console.WriteLine($"Processing PayPal payment of {amount}"); } } class Program { static void Main() { Payment payment = new CreditCardPayment(); // Abstract type, specific method payment.MakePayment(100.50m); // Using abstraction payment = new PayPalPayment(); // Switch to another implementation payment.MakePayment(75.25m); // Still using abstraction } }
Explanation:
- Abstract Class (
Payment
):- Provides a general template for all payment types (
MakePayment
method) without specifying details.
- Provides a general template for all payment types (
- Concrete Classes (
CreditCardPayment
,PayPalPayment
):- Implement the payment behavior uniquely for each payment type.
- Main Program:
- The user interacts with the
Payment
class (abstract type) without worrying about implementation details.
- The user interacts with the
Abstraction = Focusing on what something does, not how it does it.
The abstract class (Payment
) in this example provides a blueprint with a method (MakePayment
) that defines the essential behavior, but leaves the specific implementation to derived classes. The two concrete classes (CreditCardPayment
and PayPalPayment
) implement the abstract method, each providing their own details on how the payment is processed, while the user interacts only with the high-level abstraction.
Explain Inheritance with an example?
Inheritance allows a class to inherit properties and methods from another class enabling code reuse.
Online Payment Example Demonstrating Inheritance: Let’s define a base class OnlinePayment
and derive specific payment types like CreditCardPayment
and PayPalPayment
.
// Base class class OnlinePayment { public void Pay(decimal amount) { Console.WriteLine($"Payment of {amount} initiated."); } } // Derived class for Credit Card class CreditCardPayment : OnlinePayment { public void ValidateCard() { Console.WriteLine("Validating credit card details."); } } // Derived class for PayPal class PayPalPayment : OnlinePayment { public void LoginToPayPal() { Console.WriteLine("Logging in to PayPal account."); } } class Program { static void Main() { // Using CreditCardPayment CreditCardPayment creditCard = new CreditCardPayment(); creditCard.Pay(150); // Inherited method creditCard.ValidateCard(); // Specific to CreditCardPayment // Using PayPalPayment PayPalPayment payPal = new PayPalPayment(); payPal.Pay(75); // Inherited method payPal.LoginToPayPal(); // Specific to PayPalPayment } }
Explain polymorphism with real world example
Polymorphism means "many forms" and allows objects to behave differently based on their type while sharing the same interface or parent class. This helps in writing flexible and reusable code.
Real-World Example: Online Payment
In an online payment system, you might have different payment methods (like Credit Card, PayPal, etc.), but the way they process payments could differ.
// Base Class abstract class Payment { public abstract void ProcessPayment(decimal amount); // Abstract method } // Derived Class for Credit Card class CreditCardPayment : Payment { public override void ProcessPayment(decimal amount) { Console.WriteLine($"Processing credit card payment of {amount}"); } } // Derived Class for PayPal class PayPalPayment : Payment { public override void ProcessPayment(decimal amount) { Console.WriteLine($"Processing PayPal payment of {amount}"); } } // Derived Class for Bank Transfer class BankTransferPayment : Payment { public override void ProcessPayment(decimal amount) { Console.WriteLine($"Processing bank transfer payment of {amount}"); } } class Program { static void Main() { // Polymorphism in action: Same method called on different objects Payment payment; payment = new CreditCardPayment(); payment.ProcessPayment(100); payment = new PayPalPayment(); payment.ProcessPayment(200); payment = new BankTransferPayment(); payment.ProcessPayment(300); } }
Explanation:
- Base Class (
Payment
):- Defines the common interface (
ProcessPayment
method).
- Defines the common interface (
- Derived Classes:
- Implement the method differently (
CreditCardPayment
,PayPalPayment
,BankTransferPayment
).
- Implement the method differently (
- Polymorphism in Action:
- The base class type (
Payment
) is used to hold the reference to different derived class objects. - At runtime, the appropriate method is called based on the actual object type.
- The base class type (
Real-World Analogy:
Think of a "Pay Now" button on a shopping website:
- Single interface: The button performs the same action (process payment).
- Multiple implementations: The payment is processed differently based on whether you choose Credit Card, PayPal, or Bank Transfer.
Key Takeaway:
Polymorphism simplifies interactions by allowing you to write code that works with different types in a unified way. It provides flexibility by allowing method behavior to adapt dynamically at runtime.
What is the difference between method overloading and method overriding?
Method Overloading: Same method name, different parameters.
Method Overriding: A derived class changes the implementation of a method from the base class using the override keyword.
What are access modifiers in C#?
Access modifiers control the visibility of a member or class:
public, private, protected, internal, protected internal, private protected.
What is an abstract class, and how is it different from an interface?
Abstract Class: Cannot be instantiated and can have both implemented and abstract methods.
Interface: Only defines contracts (all methods are public and abstract by default) with no implementation.
What is an interface in C#?
An interface in C# is a contract that defines a set of methods, properties, events, or indexers that a class or struct must implement. It only specifies what members must be implemented, without providing any code for them (except for default methods in newer versions of C#).
What is the purpose of an interface?
Interfaces are used for:
- Achieving abstraction.
- Implementing multiple inheritance (unlike classes, where multiple inheritance is not supported).
- Creating loosely coupled designs.
- Defining shared behavior across unrelated classes.
What are the key features of an interface?
- Members in an interface are public by default.
- An interface does not contain implementation logic (except for default interface methods starting from C# 8.0).
- A class or struct can implement multiple interfaces.
- Interfaces cannot have constructors, fields, or destructors.
Can an interface inherit another interface?
Yes, an interface can inherit one or more other interfaces. This is called interface inheritance.
Example:
interface IBase { void BaseMethod(); } interface IDerived : IBase { void DerivedMethod(); }
What is the difference between an abstract class and an interface?
Abstract Class
- Can have implementation and abstract members.
- Supports single inheritance only.
- Can have fields.
- Use abstract and virtual.
Interface
- Only defines the members (until C# 8.0). From C# 8.0 interfaces can have default implementation.
- Supports multiple inheritance.
- Cannot have fields.
- All members are implicitly abstract.
What happens if a class does not implement all the methods in an interface?
The class becomes abstract and must be marked as such. It is the responsibility of derived classes to implement the remaining members.
What are default interface methods?
Introduced in C# 8.0, default methods allow an interface to provide a default implementation for a member.
Example:
interface ILogger { void Log(string message); // Abstract method // Default implementation void LogInfo(string message) { Console.WriteLine($"INFO: {message}"); } } class ConsoleLogger : ILogger { public void Log(string message) { Console.WriteLine($"LOG: {message}"); } }
Can a class explicitly implement an interface? What is explicit implementation?
A class can implement an interface explicitly by prefixing the method with the interface name.
Explicit implementation is used to:
- Avoid name conflicts when multiple interfaces define methods with the same name.
- Prevent direct access to the interface methods from the class instance.
Example:
interface IFly { void Fly(); } interface IWalk { void Walk(); } class Bird : IFly, IWalk { void IFly.Fly() { Console.WriteLine("Flying..."); } void IWalk.Walk() { Console.WriteLine("Walking..."); } } class Program { static void Main() { Bird bird = new Bird(); // Explicit implementation requires casting ((IFly)bird).Fly(); // Output: Flying... ((IWalk)bird).Walk(); // Output: Walking... } }
What is the use of the IS
keyword with interfaces?
The is
keyword checks whether an object implements a particular interface.
Example:
interface IDriveable {} class Car : IDriveable {} class Program { static void Main() { Car car = new Car(); Console.WriteLine(car is IDriveable); // Output: True } }
What is a delegate in C#?
A delegate is a type-safe function pointer in C#. It defines a reference type that can hold a reference to methods with a specific signature.
Why are delegates used in C#?
Delegates are used to:
- Allow methods to be passed as parameters.
- Enable callback mechanisms.
- Facilitate event handling in C#.
- Write flexible and extensible applications using multicast delegates.
How do you declare and use a delegate in C#?
using System; // Declare a delegate delegate void PrintMessage(string message); class Program { static void PrintToConsole(string message) { Console.WriteLine(message); } static void Main() { // Instantiate the delegate PrintMessage printer = PrintToConsole; // Invoke the delegate printer("Hello, Delegates!"); } }
What are the types of delegates in C#?
- Single-cast Delegate: Holds a reference to one method.
- Multicast Delegate: Holds references to multiple methods, invoking them in the order they are added.
What are multicast delegates?
A multicast delegate can hold references to multiple methods. When invoked, all methods in the delegate invocation list are executed sequentially.
Example:
using System; delegate void PrintMessage(string message); class Program { static void Method1(string message) { Console.WriteLine($"Method1: {message}"); } static void Method2(string message) { Console.WriteLine($"Method2: {message}"); } static void Main() { PrintMessage printer = Method1; printer += Method2; printer("Hello, Multicast Delegate!"); // Calls both Method1 and Method2 } }
What are Func
, Action
, and Predicate
delegates in C#?
Func<T, TResult>
:- Used for delegates that return a value.
- Example:
Func<int, int, int> add = (a, b) => a + b;
Action<T>
:- Used for delegates that do not return a value (
void
methods). - Example:
Action<string> print = message => Console.WriteLine(message);
- Used for delegates that do not return a value (
Predicate<T>
:- Used for delegates that return a
bool
(true/false). - Example:
Predicate<int> isEven = num => num % 2 == 0;
- Used for delegates that return a
What do you mean by a delegate is a type-safe function pointer in C#?
When we say a delegate is a type-safe function pointer in C#, it means the following:
Type-Safety in Delegates
A delegate enforces type-safety by ensuring that:
- The method assigned to the delegate must match its signature and return type.
- You cannot assign a method with a mismatched signature or return type to a delegate.
- Delegates prevent accidental assignment of methods with incompatible types, ensuring type safety at compile time.
Example of Type-Safe Behavior:
// Declare a delegate with a specific signature delegate void PrintDelegate(string message); class Program { static void PrintToConsole(string message) { Console.WriteLine(message); } static int InvalidMethod() { return 42; } static void Main() { PrintDelegate printer = PrintToConsole; // Valid - matches the signature printer("Hello, Delegates!"); // Output: Hello, Delegates! // PrintDelegate invalidPrinter = InvalidMethod; // Error: Cannot convert method 'InvalidMethod' to delegate 'PrintDelegate' (type mismatch) } }
Function Pointer Behavior
A function pointer is a reference to a method that can be invoked indirectly. In C#, delegates provide this capability with added type-safety.
Dynamic Method Assignment Example:
delegate void OperationDelegate(int x, int y); class Program { static void Add(int a, int b) { Console.WriteLine($"Sum: {a + b}"); } static void Subtract(int a, int b) { Console.WriteLine($"Difference: {a - b}"); } static void Main() { OperationDelegate operation; operation = Add; // Assign the Add method operation(10, 5); // Output: Sum: 15 operation = Subtract; // Reassign to Subtract operation(10, 5); // Output: Difference: 5 } }
This shows how a delegate allows you to reference and invoke different methods with a compatible signature dynamically at runtime.
Without type-safety, function pointers in other programming languages can lead to runtime errors if a function with an incompatible signature is called. Delegates eliminate such issues by validating the method signatures at compile-time, ensuring correctness.
Summary:
- A delegate is a function pointer because it holds a reference to a method.
- It is type-safe because it only allows methods with a signature matching the delegate's declaration, ensuring no runtime mismatch errors.
What is an Anonymous Method in C#?
An anonymous method is a method without a name, defined using the delegate keyword. It is directly assigned to a delegate variable or used inline, making code concise and improving readability. Anonymous methods were introduced in C# 2.0 and are often used for short, single-use pieces of logic.
Example of an Anonymous Method using delegate keyword:
using System; delegate void PrintDelegate(string message); class Program { static void Main() { // Anonymous method assigned to a delegate PrintDelegate printer = delegate (string message) { Console.WriteLine(message); }; printer("Hello from Anonymous Method!"); // Output: Hello from Anonymous Method! } }
We can rewrite the example using a lambda expression, which is a more modern and concise way to define anonymous methods. Lambda expressions were introduced in C# 3.0 and are often preferred for their simplicity and readability.
Example of an Anonymous Method using Lambda expression:
using System; delegate void PrintDelegate(string message); class Program { static void Main() { // Lambda expression assigned to a delegate PrintDelegate printer = message => Console.WriteLine(message); printer("Hello from Lambda Expression!"); // Output: Hello from Lambda Expression! } }
Key Changes:
- The
delegate
keyword is replaced by the=>
lambda operator. - The parameter
message
is directly followed by the expressionConsole.WriteLine(message)
, making it shorter and easier to read. - Lambda expressions are the recommended way to define inline methods in modern C#.
What is a static class in C#?
A static class is a class that:
- Cannot be instantiated (you cannot create an object of it).
- It cannot have instance constructors or destructors.
- Contains only static members (methods, properties, fields, etc.).
- Is sealed by default.
- The static modifier ensures that there is only one copy of the class, making it efficient for global state or utility methods.
How do you declare a static class in C#?
static class MathUtils { public static int Add(int x, int y) { return x + y; } } class Program { static void Main() { Console.WriteLine(MathUtils.Add(5, 10)); // Output: 15 } }
What is a static method in C#?
A static method is a method declared with the static modifier. It can be called:
- Without creating an object of the class.
- Using the class name directly.
It cannot access instance members (non-static members) of the class.
Can a static method access instance variables?
No, static methods cannot access instance variables or instance methods directly, because instance members are tied to a specific object, while static members are tied to the class itself.
Can a static class inherit or be inherited?
A static class cannot inherit from another class or be inherited by another class because inheritance is only meaningful for objects. Static classes are not instantiated and hence do not follow object-oriented inheritance.
Can a static method be overridden?
No, static methods cannot be overridden because they are not bound to an instance of the class. They are bound to the class itself at compile-time.
What are the use cases for static methods and static classes?
- Static Methods: For functionality independent of any object state.
- Example: Utility methods like
Math.Abs()
,String.Concat()
.
- Example: Utility methods like
- Static Classes: For grouping related utility functions or constants.
- Example:
System.Math
,Environment
- Example:
How is memory allocated for static members?
Static members are stored in the application domain's heap, and there is only one shared copy of static members across all instances or usages of the class.
Can a static class contain non-static members?
No, a static class can only contain static members. Non-static members require object instantiation, which is not allowed for static classes.
Can static constructors have access modifiers?
No, static constructors cannot have access modifiers. They are always private and are invoked by the runtime once, before the first use of any static member.
What is a static constructor in C#?
A static constructor:
- Initializes static fields of a class.
- Is called automatically, once, when any static member is accessed for the first time.
- Does not take parameters.
static class Logger { public static string LogPath; static Logger() { LogPath = "C:Logsapp.log"; } } class Program { static void Main() { Console.WriteLine(Logger.LogPath); // Output: C:Logsapp.log } }
Can a static class implement an interface?
No, static classes cannot implement interfaces because interfaces are intended to define behaviors that instances of classes must implement.
What are limitations of static classes?
- Cannot inherit or be inherited.
- Cannot create objects of the class.
- Limited to stateless designs.
What is the difference between a const
, readonly
, and static variable?
const
Variable: Compile-time constant that cannot change.readonly
Variable: Run-time constant that can only be assigned once (in the constructor or declaration).- Static Variable: Shared variable for the whole class and initialized once.