Python training institute in bangalore | Python online training in bangalore marathahalli
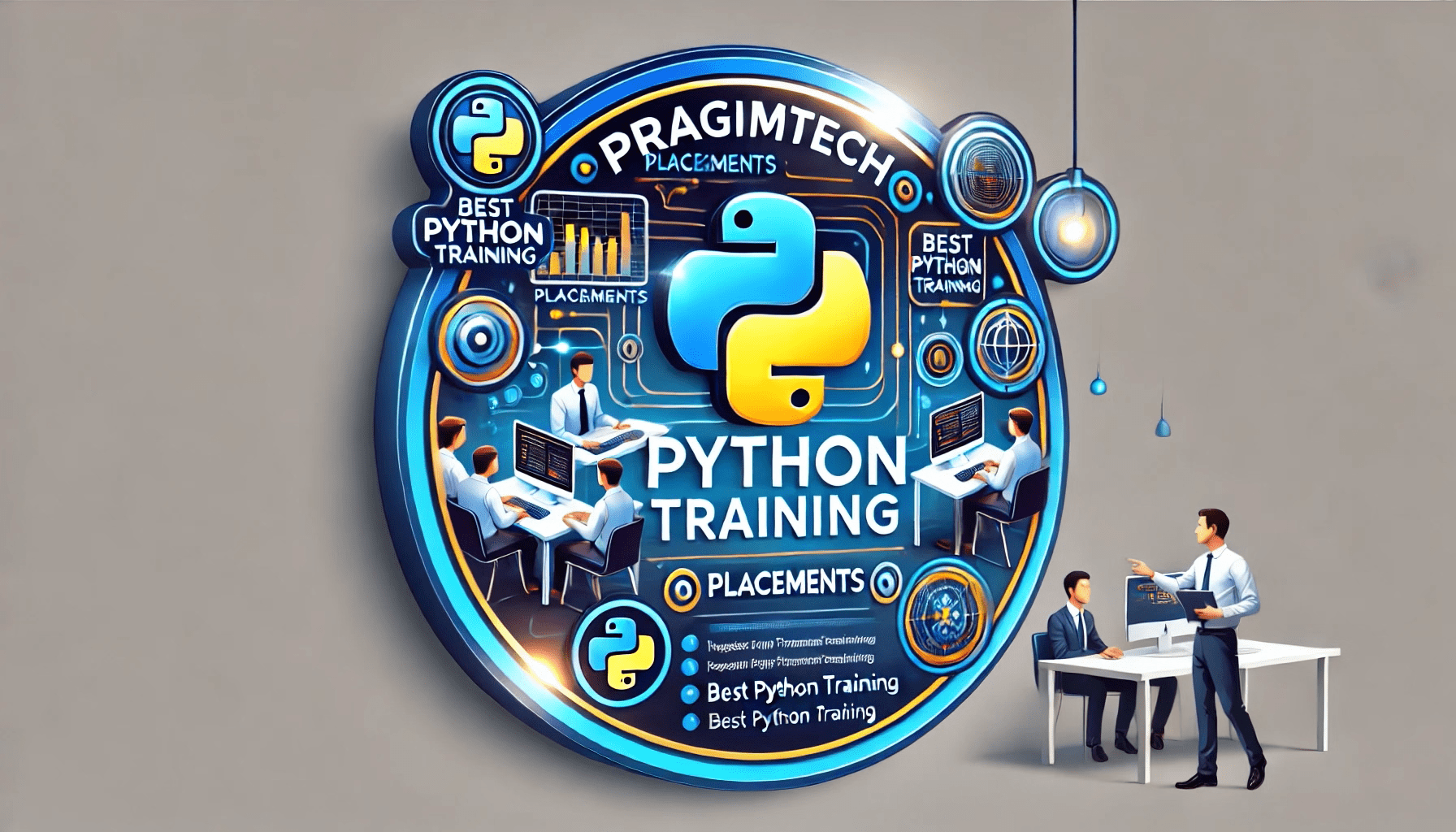
Best Python Training Institute in Bangalore | Python Online Training
PragimTech is the best Python training institute in Bangalore. We offer both classroom and online training. Our Python trainer has over 20 years experience and we have place over 3000 students in 2024 alone in different companies like IBM, Dell, Microsoft, Mercer, HCL, TCS. Training covers everything you need from the basics. Regarding placements please speak to our previous batch students. To join our course please call us on +91 99456 99393 or pragim@gmail.com
Python interview questions and answers
What are variables in Python? How are they declared?
Variables in Python are used to store data values. Unlike other programming languages, variables in Python do not require explicit declaration of their data types, they are dynamically typed.
# Example name = "Alice" # String age = 25 # Integer height = 5.5 # Float
What are the common data types in Python?
Python has the following common data types:
- Numeric: int, float, complex
- Text: str
- Sequence: list, tuple, range
- Mapping: dict
- Set types: set, frozenset
- Boolean: bool
- Binary: bytes, bytearray, memoryview
How can you take input from a user in Python?
You can use the input()
function to take user input as a string. If you need numeric input, cast it to int
or float
.
name = input("Enter your name: ") age = int(input("Enter your age: ")) print(f"Hello {name}, you are {age} years old.")
How do you display output in Python?
You can use the print()
function to display output.
print("Hello, World!") name = "Alice" print(f"My name is {name}.")
Why is Python called an "interpreted language"?
Python is called an interpreted language because its code is executed line by line at runtime without needing to compile it into machine-level code beforehand.
How does indentation work in Python?
Python uses indentation to define blocks of code, such as within loops, conditionals, and functions. Indentation is mandatory in Python, unlike in some other languages where braces {} or end keywords are used.
# Correct example if 5 > 2: print("Five is greater than two") # Incorrect (missing indentation) # if 5 > 2: # print("Five is greater than two")
What happens if you use inconsistent indentation in Python?
Python will throw an IndentationError
. For example:
# Code with inconsistent indentation if True: print("Hello") print("World") # This line has inconsistent spacing # Output: IndentationError: unexpected indent
What are comments in Python, and why are they used?
Comments are used to describe the purpose of code or leave notes for other developers. In Python:
Single-line comments begin with #
Multi-line comments can use triple quotes ('''
or """
)
# Single-line comment ''' This is a multi-line comment. '''
Can you provide an example of multi-line documentation?
Multi-line documentation is usually placed at the start of scripts, functions, or classes using triple quotes.
def add(a, b): """ This function adds two numbers. Parameters: a (int): First number b (int): Second number Returns: int: Sum of a and b """ return a + b
How does Python treat variables of different types? Can you change a variable's type?
Python allows a variable to change its type dynamically. For example:
x = 10 # Integer x = "Hello" # Now a string