C Sharp tutorial for beginners
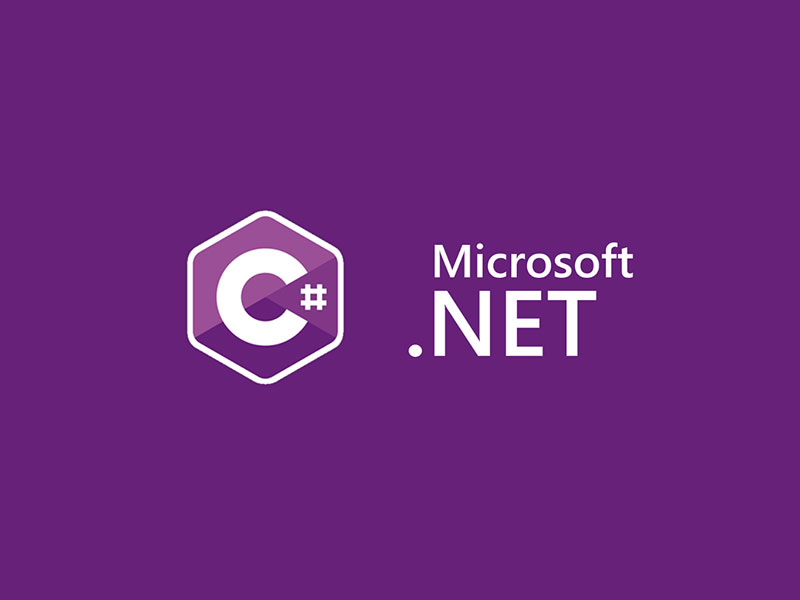
Free .NET Training Videos for beginners and intermediate programmers
For your convenience, we have arranged all the Dot Net basics, C# and SQL Server videos in a logical sequence using youtube playlists. If you would like to watch the videos directly on youtube, click on the links below. We hope you enjoy these videos. Happy learning. We want these videos to be helpful for as many people as possible. Please feel free to share the links with your friends and family.
Dot Net Basics Video Tutorial Playlist
SQL Server video tutorial playlist
Subscribe to receive an email, when new videos are uploaded
Free DotNet Basic Videos
- Text | Slides | Dot Net Program Execution
- Text | Slides | ILDASM and ILASM
- Text | Slides | Strong naming an assembly
- Text | Slides | GAC
- Text | Slides | How .NET finds the assemblies
- Text | Slides | DLL Hell
- Text | Slides | DLL Hell solution
Free C# Video Tutorials
- Text | Slides | Introduction
- Text | Slides | Reading and writing to a console
- Text | Slides | Built-in data types
- Text | Slides | String data type
- Text | Slides | Operators
- Text | Slides | Nullable Types
- Text | Slides | Datatype conversions
- Text | Slides | Arrays
- Text | Slides | Comments
- Text | Slides | If statement
- Text | Slides | Switch statement
- Text | Slides | Switch continued
- Text | Slides | While loop
- Text | Slides | Do while loop
- Text | Slides | For & foreach loop
- Text | Slides | Methods
- Text | Slides | Method parameters
- Text | Slides | Namespaces
- Text | Slides | Class - Introduction
- Text | Slides | Static & Instance members
- Text | Slides | Inheritance
- Text | Slides | Method hiding
- Text | Slides | Polymorphism
- Text | Slides | Method overriding Vs hiding
- Text | Slides | Method overloading
- Text | Slides | Why Properties
- Text | Slides | Properties
- Text | Slides | Structs
- Text | Slides | Classes Vs Structs
- Text | Slides | Interfaces
- Text | Slides | Explicit interface implementation
- Text | Slides | Abstract Classes
- Text | Slides | Abstract Classes Vs Interfaces
- Text | Slides | Diamond Problem
- Text | Slides | Multiple inheritance
- Text | Slides | Delegates
- Text | Slides | Delegates Example - I
- Text | Slides | Delegates Example - II
- Text | Slides | Multicast Delegates
- Text | Slides | Exception Handling
- Text | Slides | Inner Exceptions
- Text | Slides | Custom Exceptions
- Text | Slides | Exception Handling Abuse
- Text | Slides | Preventing Exception Handling Abuse
- Text | Slides | Why Enums
- Text | Slides | Enums Example
- Text | Slides | Enums Concepts
- Text | Slides | Types v/s Type Members
- Text | Slides | Access Modifiers - Private, Public and Protected
- Text | Slides | Access Modifiers - Internal and Protected Internal
- Text | Slides | Access Modifiers for types
- Text | Slides | Attributes
- Text | Slides | Reflection
- Text | Slides | Reflection Example
- Text | Slides | Late binding using reflection
- Text | Slides | Generics
- Text | Slides | Reason to override ToString() method
- Text | Slides | Reason to override Equals() method
- Text | Slides | Difference between Convert.ToString() and ToString() method
- Text | Slides | Difference between string and stringbuilder
- Text | Slides | Partial classes in C#
- Text | Slides | Creating partial classes in C#
- Text | Slides | Partial methods in c# - Part 63
- Text | Slides | How and where are indexers used in .net
- Text | Slides | Indexers in c#
- Text | Slides | Overloading indexers
- Text | Slides | Optional parameters
- Text | Slides | Making method parameters optional using method overloading
- Text | Slides | Making method parameters optional by specifying parameter defaults
- Text | Slides | Making method parameters optional by using OptionalAttribute
- Text | Slides | Code snippets in visual studio
- Text | Slides | What is dictionary in c#
- Text | Slides | What is dictionary in c# continued
- Text | Slides | List collection class in c#
- Text | Slides | List collection class in c# continued
- Text | Slides | Working with generic list class and ranges in c#
- Text | Slides | Sort a list of simple types in c#
- Text | Slides | Sort a list of complex types in c#
- Text | Slides | Sort a list of complex types using Comparison delegate
- Text | Slides | Some useful methods of List collection class
- Text | Slides | When to use a dictionary over list in c#
- Text | Slides | Generic queue collection class
- Text | Slides | Generic stack collection class
- Text | Slides | Real time example of queue collection class in c#
- Text | Slides | Real time example of stack collection class in c#
- Text | Slides | Multithreading in C#
- Text | Slides | Advantages and disadvantages of multithreading
- Text | Slides | ThreadStart delegate
- Text | Slides | ParameterizedThreadStart delegate
- Text | Slides | Passing data to the Thread function in a type safe manner
- Text | Slides | Retrieving data from Thread function using callback method
- Text | Slides | Significance of Thread.Join and Thread.IsAlive functions
- Text | Slides | Protecting shared resources from concurrent access in multithreading
- Text | Slides | Difference between Monitor and lock in C#
- Text | Slides | Deadlock in a multithreaded program
- Text | Slides | How to resolve a deadlock in a multithreaded program
- Text | Slides | Performance of a multithreaded program
- Text | Slides | Anonymous methods in c#
- Text | Slides | Lambda expression in c#
- Text | Slides | Func delegate in c#
- Text | Slides | Async and await in c#
- Text | Slides | How to wait for a thread to finish without blocking