Python training institute in bangalore | Python online training in bangalore marathahalli
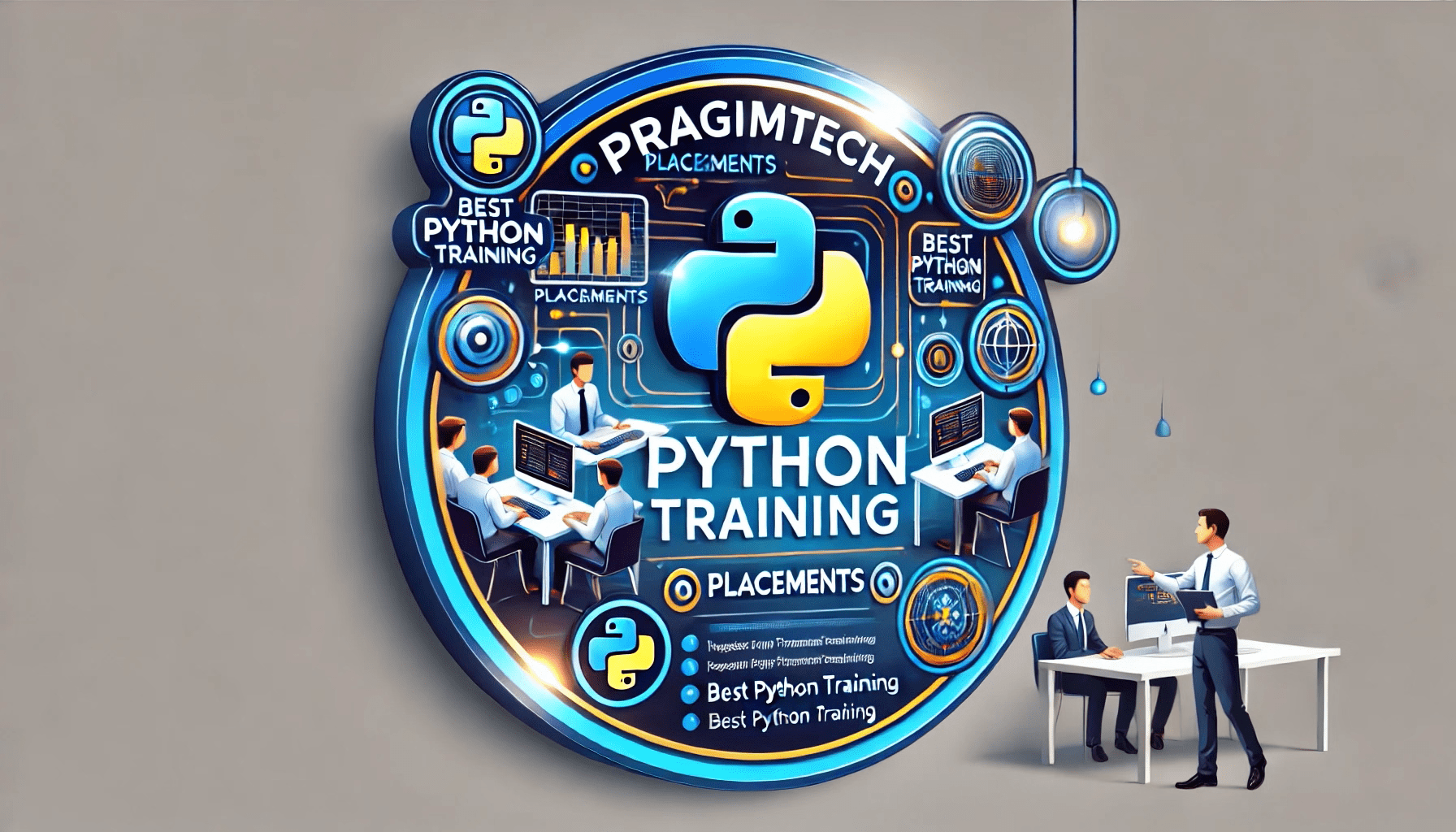
Best Python Training Institute in Bangalore | Python Online Training
PragimTech is the best Python training institute in Bangalore. We offer both classroom and online training. Our Python trainer has over 20 years experience and we have place over 3000 students in 2024 alone in different companies like IBM, Dell, Microsoft, Mercer, HCL, TCS. Training covers everything you need from the basics. Regarding placements please speak to our previous batch students. To join our course please call us on +91 99456 99393 or pragim@gmail.com
Python interview questions and answers
What are variables in Python? How are they declared?
Variables in Python are used to store data values. Unlike other programming languages, variables in Python do not require explicit declaration of their data types, they are dynamically typed.
# Example name = "Alice" # String age = 25 # Integer height = 5.5 # Float
What are the common data types in Python?
Python has the following common data types:
- Numeric: int, float, complex
- Text: str
- Sequence: list, tuple, range
- Mapping: dict
- Set types: set, frozenset
- Boolean: bool
- Binary: bytes, bytearray, memoryview
How can you take input from a user in Python?
You can use the input()
function to take user input as a string. If you need numeric input, cast it to int
or float
.
name = input("Enter your name: ") age = int(input("Enter your age: ")) print(f"Hello {name}, you are {age} years old.")
How do you display output in Python?
You can use the print()
function to display output.
print("Hello, World!") name = "Alice" print(f"My name is {name}.")
Why is Python called an "interpreted language"?
Python is called an interpreted language because its code is executed line by line at runtime without needing to compile it into machine-level code beforehand.
How does indentation work in Python?
Python uses indentation to define blocks of code, such as within loops, conditionals, and functions. Indentation is mandatory in Python, unlike in some other languages where braces {} or end keywords are used.
# Correct example if 5 > 2: print("Five is greater than two") # Incorrect (missing indentation) # if 5 > 2: # print("Five is greater than two")
What happens if you use inconsistent indentation in Python?
Python will throw an IndentationError
. For example:
# Code with inconsistent indentation if True: print("Hello") print("World") # This line has inconsistent spacing # Output: IndentationError: unexpected indent
What are comments in Python, and why are they used?
Comments are used to describe the purpose of code or leave notes for other developers. In Python:
Single-line comments begin with #
Multi-line comments can use triple quotes ('''
or """
)
# Single-line comment ''' This is a multi-line comment. '''
Can you provide an example of multi-line documentation?
Multi-line documentation is usually placed at the start of scripts, functions, or classes using triple quotes.
def add(a, b): """ This function adds two numbers. Parameters: a (int): First number b (int): Second number Returns: int: Sum of a and b """ return a + b
How does Python treat variables of different types? Can you change a variable's type?
Python allows a variable to change its type dynamically. For example:
x = 10 # Integer x = "Hello" # Now a string
Explain mutable and immutable data types in Python with examples.
Mutable: Can be changed after creation (e.g., list
, dict
)
Immutable: Cannot be changed after creation (e.g., str
, tuple
)
# Mutable example my_list = [1, 2, 3] my_list.append(4) # List is modified # Immutable example my_tuple = (1, 2, 3) # my_tuple[0] = 0 # Throws a TypeError
How does Python handle case sensitivity?
Python is case-sensitive. For example, Name
and name
are considered different identifiers.
How can you check the type of a variable in Python?
You can use the type()
function.
x = 10 print(type(x)) # <class 'int'>
Can you assign multiple variables in a single line?
Yes, Python allows multiple assignments in a single line.
x, y, z = 1, "Hello", 3.14
What is the difference between is
and ==
in Python?
== checks for value equality.
is checks for reference equality (whether two objects are the same in memory).
a = [1, 2, 3] b = [1, 2, 3] print(a == b) # True (values are equal) print(a is b) # False (different objects)
Can Python variables have special characters?
Variable names can include letters, digits, and underscores (_) but cannot start with a digit or include special characters like @
, $
, etc.
What will happen if you try to access an undefined variable in Python?
You’ll get a NameError
print(x) # NameError: name 'x' is not defined
What is the difference between for
and while
loops in Python?
A for
loop iterates over a sequence (like a list
, tuple
, or range
).
A while
loop continues until its condition evaluates to False
.
# For loop example for i in range(5): print(i) # While loop example i = 0 while i < 5: print(i) i += 1
How does the range()
function work in a for
loop?
The range()
function generates a sequence of numbers.
range(stop) → Starts at 0, ends at stop - 1. range(start, stop, step) → Custom start, stop, and step values. for i in range(1, 10, 2): # Outputs: 1, 3, 5, 7, 9 print(i)
Can you nest loops in Python? Provide an example.
Yes, loops can be nested in Python. This means placing one loop inside another, where the inner loop is executed for every iteration of the outer loop.
# Nested loops example for i in range(3): for j in range(2): print(f"i={i}, j={j}")
# Output: # i=0, j=0 # i=0, j=1 # i=1, j=0 # i=1, j=1 # i=2, j=0 # i=2, j=1
What does the break
statement do in a loop
? Provide an example.
The break
statement terminates the loop
immediately.
for i in range(5): if i == 3: break print(i) # Output: 0, 1, 2
What is the purpose of the continue
statement? Provide an example.
The continue
statement skips the current iteration and moves to the next.
for i in range(5): if i == 2: continue print(i) # Output: 0, 1, 3, 4
What is the pass
statement used for? Provide an example.
The pass
statement in Python does nothing when executed; it acts as a placeholder to ensure that the code runs without errors where a block of code is required syntactically but you don’t want to implement logic yet. It’s often used in scenarios where the structure of the code is being set up but the actual logic will be written later.
for i in range(5): # Loop through numbers 0 to 4 if i == 2: pass # Placeholder for future logic print(i) # Prints the number
What happens if the break
statement is used inside a nested loop?
The break
statement will terminate the innermost loop where it’s used.
for i in range(3): for j in range(3): if j == 1: break print(f"i={i}, j={j}")
Output: i=0, j=0 i=1, j=0 i=2, j=0
How can you use an else
clause with a loop?
The else
block after a loop runs if the loop completes without encountering a break
.
for i in range(5): if i == 3: break else: print("Completed") # Output: (No output as break is encountered) for i in range(5): if i == 6: # This condition will not trigger break else: print("Completed") # Output: Completed
How do infinite loops occur in Python, and how can they be avoided?
Infinite loops occur when the condition in a while loop never evaluates to False
. This causes the loop to run indefinitely, potentially freezing the program or causing it to consume excessive resources. To avoid infinite loops, ensure the loop condition changes during each iteration so that it eventually becomes False
.
# Infinite loop example count = 0 while count < 5: print(count) # The condition is always True because count is never updated # To terminate: Use Ctrl+C or stop the script. # Loop that eventually finishes count = 0 while count < 5: print(count) # Output the current value of count count += 1 # Increment count to ensure the condition eventually becomes False
How can you iterate through both keys
and values
in a dictionary
using a for
loop?
Use the items()
method to iterate through key-value pairs.
my_dict = {'a': 1, 'b': 2, 'c': 3} for key, value in my_dict.items(): print(f"{key}: {value}")
Output: a: 1 b: 2 c: 3
What is the difference between a list
and a tuple
in Python?
A list
is mutable, meaning its elements can be changed after creation.
A tuple
is immutable, meaning its elements cannot be modified after creation.
# List (Mutable) my_list = [1, 2, 3] my_list[0] = 10 # Allowed # Tuple (Immutable) my_tuple = (1, 2, 3) # my_tuple[0] = 10 # TypeError: 'tuple' object does not support item assignment
What is a list, and how is it different from an array?
A list in Python is a collection that is:
- Ordered: Elements have a specific order.
- Mutable: Can be modified (add, remove, change elements).
- Heterogeneous: Can store different data types.
Difference from arrays:
- Lists can store different data types, while arrays (from the array module) store only one type of data.
- Lists are more flexible, but arrays are more memory-efficient for large numerical data.
my_list = [1, "hello", 3.14, True] # A list with mixed data types
How do you remove duplicate elements from a list?
You can use the set() function or a loop to remove duplicates while maintaining order.
Method 1: Using set()
(Unordered result)
my_list = [1, 2, 2, 3, 4, 4, 5] unique_list = list(set(my_list)) print(unique_list) # Output may vary (e.g., [1, 2, 3, 4, 5])
Method 2: Using a loop
(Maintains order)
my_list = [1, 2, 2, 3, 4, 4, 5] unique_list = [] for num in my_list: if num not in unique_list: unique_list.append(num) print(unique_list) # Output: [1, 2, 3, 4, 5]
How do you reverse a list in Python?
There are multiple ways to reverse a list:
Method 1: Using slicing
my_list = [1, 2, 3, 4, 5] reversed_list = my_list[::-1] print(reversed_list) # Output: [5, 4, 3, 2, 1]
Method 2: Using reverse()
(Modifies the list in place)
my_list = [1, 2, 3, 4, 5] my_list.reverse() print(my_list) # Output: [5, 4, 3, 2, 1]
Method 3: Using reversed()
(Returns an iterator)
my_list = [1, 2, 3, 4, 5] reversed_list = list(reversed(my_list)) print(reversed_list) # Output: [5, 4, 3, 2, 1]
How do you merge two lists in Python?
There are several ways to merge two lists:
Method 1: Using +
operator
list1 = [1, 2, 3] list2 = [4, 5, 6] merged_list = list1 + list2 print(merged_list) # Output: [1, 2, 3, 4, 5, 6]
Method 2: Using extend()
(Modifies the first list in place)
list1 = [1, 2, 3] list2 = [4, 5, 6] list1.extend(list2) print(list1) # Output: [1, 2, 3, 4, 5, 6]
Method 3: Using itertools.chain()
(Efficient for large lists)
import itertools list1 = [1, 2, 3] list2 = [4, 5, 6] merged_list = list(itertools.chain(list1, list2)) print(merged_list) # Output: [1, 2, 3, 4, 5, 6]
How do you find the most frequent element in a list?
You can use collections.Counter
or a loop.
Method 1: Using Counter
(Best for large lists)
from collections import Counter my_list = [1, 2, 2, 3, 3, 3, 4, 4, 4, 4] most_common = Counter(my_list).most_common(1)[0][0] print(most_common) # Output: 4
Method 2: Using a loop
(For smaller lists)
my_list = [1, 2, 2, 3, 3, 3, 4, 4, 4, 4] freq_dict = {} for num in my_list: freq_dict[num] = freq_dict.get(num, 0) + 1 most_common = max(freq_dict, key=freq_dict.get) print(most_common) # Output: 4
How do you add and remove elements in a list?
Use append()
, insert()
, or extend()
to add elements.
Use remove()
, pop()
, or del
to remove elements.
my_list = [1, 2, 3] # Adding elements my_list.append(4) # [1, 2, 3, 4] my_list.insert(1, 10) # [1, 10, 2, 3, 4] my_list.extend([5, 6]) # [1, 10, 2, 3, 4, 5, 6] # Removing elements my_list.remove(10) # [1, 2, 3, 4, 5, 6] my_list.pop(2) # Removes element at index 2: [1, 2, 4, 5, 6] del my_list[0] # Deletes first element: [2, 4, 5, 6]
What is a tuple, and how is it different from a list?
A tuple is an immutable sequence in Python, meaning its elements cannot be changed after creation. It is defined using parentheses ()
and can hold heterogeneous data types.
Difference between List and Tuple:
- List: Mutable, defined using
[]
, slower due to extra memory usage. - Tuple: Immutable, defined using
()
, faster and consumes less memory.
my_list = [1, 2, 3] # List my_tuple = (1, 2, 3) # Tuple my_list[0] = 100 # Allowed # my_tuple[0] = 100 # TypeError: 'tuple' object does not support item assignment
How can you create a tuple with a single element?
To create a single-element tuple, a trailing comma ,
must be included; otherwise, Python treats it as a regular variable.
single_element_tuple = (10,) # Tuple with one element not_a_tuple = (10) # Just an integer print(type(single_element_tuple)) # Output: <class 'tuple'> print(type(not_a_tuple)) # Output: <class 'int'>
How do you access elements in a tuple?
Elements in a tuple can be accessed using indexing
(positive and negative) and slicing
.
tup = (10, 20, 30, 40, 50) print(tup[0]) # Output: 10 (First element) print(tup[-1]) # Output: 50 (Last element) print(tup[1:4]) # Output: (20, 30, 40) (Slice from index 1 to 3)
Can a tuple contain mutable objects?
Yes, a tuple itself is immutable, but it can contain mutable objects like lists.
tup = (1, 2, [3, 4]) tup[2][0] = 100 # Allowed since list inside tuple is mutable print(tup) # Output: (1, 2, [100, 4]) # However, the tuple itself cannot be modified, so tup[2] = [5, 6] would raise an error.
How can you concatenate and repeat tuples?
Tuples support concatenation using +
and repetition using *
.
tup1 = (1, 2, 3) tup2 = (4, 5, 6) concat_tup = tup1 + tup2 # (1, 2, 3, 4, 5, 6) repeat_tup = tup1 * 2 # (1, 2, 3, 1, 2, 3) print(concat_tup) print(repeat_tup)
How can you convert a list to a tuple and vice versa?
You can convert a list to a tuple using the tuple()
function and a tuple to a list using the list()
function.
my_list = [1, 2, 3, 4] my_tuple = tuple(my_list) # Convert list to tuple print(my_tuple) # Output: (1, 2, 3, 4) new_list = list(my_tuple) # Convert tuple to list print(new_list) # Output: [1, 2, 3, 4]
What is a Python dictionary, and how does it work?
A dictionary in Python is an unordered, mutable collection that stores data in key-value pairs. It is defined using curly braces {}
or the dict()
constructor. Keys must be unique and immutable (e.g., strings, numbers, tuples), while values can be of any data type.
# Creating a dictionary student = {"name": "Alice", "age": 25, "grade": "A"} # Accessing values print(student["name"]) # Output: Alice
How do you add, update, and delete elements in a dictionary?
- Add/Update: Use the assignment operator
dict[key] = value
. - Delete: Use
del dict[key]
orpop()
.
person = {"name": "John", "age": 30} # Adding a new key-value pair person["city"] = "New York" # Updating a value person["age"] = 31 # Deleting a key-value pair del person["city"] # Using pop() age = person.pop("age") print(person) # Output: {'name': 'John'} print(age) # Output: 31
How do you iterate over a dictionary?
You can iterate over keys, values, or both using for
loops.
data = {"a": 1, "b": 2, "c": 3} # Iterating over keys for key in data: print(key, end=" ") # Output: a b c # Iterating over values for value in data.values(): print(value, end=" ") # Output: 1 2 3 # Iterating over key-value pairs for key, value in data.items(): print(f"{key}: {value}") # Output: # a: 1 # b: 2 # c: 3
What are dictionary comprehensions, and how are they useful?
A dictionary comprehension is a concise way to create dictionaries using a single line of code.
# Squaring numbers from 1 to 5 squares = {x: x**2 for x in range(1, 6)} print(squares) # Output: {1: 1, 2: 4, 3: 9, 4: 16, 5: 25}
How do you merge two dictionaries in Python?
You can use the update()
method or the |
operator (Python 3.9+).
dict1 = {"a": 1, "b": 2} dict2 = {"b": 3, "c": 4} # Using update() merged_dict = dict1.copy() merged_dict.update(dict2) # Using | operator (Python 3.9+) merged_dict2 = dict1 | dict2 print(merged_dict) # Output: {'a': 1, 'b': 3, 'c': 4} print(merged_dict2) # Output: {'a': 1, 'b': 3, 'c': 4}
What is a set in Python, and how is it different from a list?
A set is an unordered collection of unique elements in Python. Unlike lists, sets do not allow duplicate values and do not maintain order.
# Creating a set my_set = {1, 2, 3, 4, 4, 5} print(my_set) # Output: {1, 2, 3, 4, 5} (Duplicates are removed) # Creating a list my_list = [1, 2, 3, 4, 4, 5] print(my_list) # Output: [1, 2, 3, 4, 4, 5] (Duplicates are allowed)
How do you perform union, intersection, and difference operations on sets?
Python provides built-in methods for these operations:
- Union (
|
orunion()
): Combines all unique elements from both sets. - Intersection (
&
orintersection()
): Returns common elements from both sets. - Difference (
-
ordifference()
): Returns elements that are in one set but not in the other.
A = {1, 2, 3, 4} B = {3, 4, 5, 6} print(A | B) # Union: {1, 2, 3, 4, 5, 6} print(A & B) # Intersection: {3, 4} print(A - B) # Difference (A - B): {1, 2} print(B - A) # Difference (B - A): {5, 6}
How can you check if a set is a subset or superset of another set?
- Subset (
issubset()
): Checks if all elements of one set exist in another set. - Superset (
issuperset()
): Checks if one set contains all elements of another set.
A = {1, 2, 3} B = {1, 2, 3, 4, 5} print(A.issubset(B)) # True (A is a subset of B) print(B.issuperset(A)) # True (B is a superset of A)
How do you remove and add elements in a set?
- add(x): Adds an element x to the set.
- remove(x): Removes x from the set (raises an error if x is not found).
- discard(x): Removes x from the set (does not raise an error if x is not found).
- pop(): Removes and returns an arbitrary element from the set.
my_set = {1, 2, 3} my_set.add(4) # {1, 2, 3, 4} my_set.remove(2) # {1, 3, 4} my_set.discard(5) # No error, set remains {1, 3, 4} popped_item = my_set.pop() # Removes an arbitrary element print(my_set)
How can you find symmetric differences between two sets?
The symmetric difference returns elements that are in either of the sets but not in both.
Using ^
operator or symmetric_difference()
method.
A = {1, 2, 3, 4} B = {3, 4, 5, 6} print(A ^ B) # Output: {1, 2, 5, 6} (elements in either A or B, but not both)
What are nested structures in Python?
Nested structures in Python refer to data structures that contain other data structures inside them. Examples include:
- A list inside a list ([[1, 2], [3, 4]])
- A dictionary inside a dictionary ({'a': {'b': 1}})
- A combination of lists, tuples, and dictionaries ({'key': [1, (2, 3), {'a': 4}]})
How do you access elements in a nested list?
You can access elements in a nested list using multiple indices.
nested_list = [[10, 20], [30, 40, [50, 60]]] print(nested_list[0][1]) # Output: 20 print(nested_list[1][2][0]) # Output: 50
How do you iterate over a nested dictionary?
You can use a recursive function or nested loops to iterate over a nested dictionary.
nested_dict = { 'user1': {'name': 'Alice', 'age': 25}, 'user2': {'name': 'Bob', 'age': 30} } for key, value in nested_dict.items(): print(f"{key}:") for sub_key, sub_value in value.items(): print(f" {sub_key} -> {sub_value}")
Output:
user1: name -> Alice age -> 25 user2: name -> Bob age -> 30
How do you update values in a nested dictionary?
You can update values using key indexing.
nested_dict = {'user': {'name': 'Alice', 'age': 25}} nested_dict['user']['age'] = 26 print(nested_dict)
Output:
{'user': {'name': 'Alice', 'age': 26}}
How do you flatten a nested list?
You can use recursion or list comprehension.
(Using Recursion):
def flatten_list(nested_list): flat_list = [] for item in nested_list: if isinstance(item, list): flat_list.extend(flatten_list(item)) else: flat_list.append(item) return flat_list nested_list = [1, [2, [3, 4]], 5] print(flatten_list(nested_list)) # Output: [1, 2, 3, 4, 5]
How do you search for a key in a nested dictionary?
Use recursion to search for a key in a deeply nested dictionary.
def search_key(nested_dict, key): if key in nested_dict: return nested_dict[key] for value in nested_dict.values(): if isinstance(value, dict): result = search_key(value, key) if result is not None: return result return None nested_dict = {'a': {'b': {'c': 42}}} print(search_key(nested_dict, 'c')) # Output: 42
How do you convert a nested dictionary to JSON?
You can use Python’s json
module.
import json nested_dict = {'user': {'name': 'Alice', 'age': 25}} json_data = json.dumps(nested_dict, indent=2) print(json_data)
Output:
json { "user": { "name": "Alice", "age": 25 } }
How do you merge two nested dictionaries?
Use recursion to merge two nested dictionaries.
def merge_dicts(dict1, dict2): for key, value in dict2.items(): if key in dict1 and isinstance(dict1[key], dict) and isinstance(value, dict): merge_dicts(dict1[key], value) else: dict1[key] = value return dict1 dict1 = {'a': {'b': 1}} dict2 = {'a': {'c': 2}, 'd': 3} print(merge_dicts(dict1, dict2))
Output:
{'a': {'b': 1, 'c': 2}, 'd': 3}
How do you find the depth of a nested list?
Use recursion to calculate the depth.
def find_depth(nested_list): if isinstance(nested_list, list): return 1 + max((find_depth(item) for item in nested_list), default=0) return 0 nested_list = [1, [2, [3, 4]], 5] print(find_depth(nested_list)) # Output: 3
How do you remove a key from a nested dictionary?
Use recursion to find and delete a key.
def remove_key(nested_dict, key): if key in nested_dict: del nested_dict[key] else: for value in nested_dict.values(): if isinstance(value, dict): remove_key(value, key) nested_dict = {'a': {'b': {'c': 42, 'd': 99}}} remove_key(nested_dict, 'c') print(nested_dict)
Output:
{'a': {'b': {'d': 99}}}
What is list comprehension in Python? How is it different from a for-loop?
List comprehension is a concise way to create lists in Python. It is faster and requires fewer lines of code compared to a traditional for loop.
List comprehension improves readability and performance.
Using a for-loop:
numbers = [] for i in range(5): numbers.append(i * i) print(numbers) # Output: [0, 1, 4, 9, 16]
Using list comprehension:
numbers = [i * i for i in range(5)] print(numbers) # Output: [0, 1, 4, 9, 16]
What is set comprehension? How is it different from list comprehension?
Set comprehension is similar to list comprehension but creates a set instead of a list. A set contains only unique values, meaning duplicate elements are automatically removed.
Using list comprehension:
nums = [1, 2, 2, 3, 4, 4, 5] list_comp = [i for i in nums] print(list_comp) # Output: [1, 2, 2, 3, 4, 4, 5]
Using set comprehension:
set_comp = {i for i in nums} print(set_comp) # Output: {1, 2, 3, 4, 5} # Key difference: A list keeps duplicates, while a set removes them.
How do you use dictionary comprehension in Python?
Dictionary comprehension is used to create dictionaries in a single line of code. The syntax follows {key: value for item in iterable}
.
Creating a dictionary with squares of numbers:
squares = {i: i * i for i in range(1, 6)} print(squares) # Output: {1: 1, 2: 4, 3: 9, 4: 16, 5: 25} # It efficiently generates a dictionary without using a loop.
Can you use conditions inside comprehensions? Provide an example.
Yes, conditions can be included in comprehensions using if statements.
Filtering even numbers using list comprehension:
even_numbers = [i for i in range(10) if i % 2 == 0] print(even_numbers) # Output: [0, 2, 4, 6, 8]
Filtering even numbers using dictionary comprehension:
even_squares = {i: i * i for i in range(10) if i % 2 == 0} print(even_squares) # Output: {0: 0, 2: 4, 4: 16, 6: 36, 8: 64} # The if condition filters values before adding them to the collection.
What is a nested comprehension? Provide an example.
A nested comprehension is when one comprehension is inside another. It is used for multi-dimensional structures.
Creating a 3x3 matrix using nested list comprehension:
matrix = [[i * j for j in range(1, 4)] for i in range(1, 4)] print(matrix) # Output: [[1, 2, 3], [2, 4, 6], [3, 6, 9]] # Each inner comprehension generates a list, and the outer comprehension collects them into a larger list.
How do you define and call a function in Python?
A function is defined using the def
keyword, and it is called by using its name followed by parentheses.
def greet(): return "Hello, World!" print(greet()) # Calling the function
Output:
Hello, World!
What is the difference between parameters and arguments?
- Parameters are variables listed in the function definition.
- Arguments are values passed when calling the function.
def add(a, b): # 'a' and 'b' are parameters return a + b print(add(5, 3)) # '5' and '3' are arguments
Output:
8
Can a function return more than one value? If yes, how?
Yes, a function can return multiple values using a tuple
.
def math_operations(a, b): return a + b, a - b, a * b, a / b result = math_operations(10, 2) print(result) # (12, 8, 20, 5.0) # Unpacking values sum_, diff, product, quotient = result print(sum_, diff, product, quotient)
Output:
(12, 8, 20, 5.0) 12 8 20 5.0
What are positional arguments in Python functions?
Positional arguments are passed in the same order as defined in the function.
def divide(a, b): return a / b print(divide(10, 2)) # 5.0
Output:
5.0
What are keyword arguments in Python?
Keyword arguments are passed using parameter names, allowing flexibility in order.
def introduce(name, age): return f"My name is {name} and I am {age} years old." print(introduce(age=30, name="Alice")) # Order does not matter
Output:
My name is Alice and I am 30 years old.
What are default arguments in Python functions?
A default argument provides a predefined value when no argument is passed.
def greet(name="Guest"): return f"Hello, {name}!" print(greet()) # Uses default value print(greet("Bob")) # Overrides default value
Output:
Hello, Guest! Hello, Bob!
What is *args in Python functions?*args
allows a function to accept any number of positional arguments.
def total(*numbers): return sum(numbers) print(total(1, 2, 3, 4)) # 10 print(total(10, 20)) # 30
Output:
10 30
What is **kwargs in Python functions?**kwargs
allows a function to accept any number of keyword arguments.
def print_details(**kwargs): for key, value in kwargs.items(): print(f"{key}: {value}") print_details(name="Alice", age=25, city="NY")
Output:
name: Alice age: 25 city: NY
Can *args and **kwargs be used together?
Yes, *args
(positional arguments) and **kwargs
(keyword arguments) can be used together in a function.
def show_data(*args, **kwargs): print("Positional arguments:", args) print("Keyword arguments:", kwargs) show_data(1, 2, 3, name="Alice", age=30)
Output:
Positional arguments: (1, 2, 3) Keyword arguments: {'name': 'Alice', 'age': 30}
What is function recursion, and how does it work in Python?
Recursion is a process where a function calls itself to solve a problem in smaller steps. It is useful for problems like calculating factorials, Fibonacci sequences, and tree traversals.
Factorial using recursion
def factorial(n): if n == 1: # Base case (stopping condition) return 1 return n * factorial(n - 1) # Recursive call print(factorial(5)) # 120
Output:
120 # Here, factorial(5) calls factorial(4), which calls factorial(3), and so on, until it reaches factorial(1), the base case.
What is a return value in Python, and why is it important?
A return
value is the output of a function that is sent back to the caller. It allows functions to process data and provide results, which can be used later in the program.
def add(a, b): return a + b # Returning the sum of a and b result = add(5, 3) print(result) # Output: 8
Can a function return multiple values in Python? How?
Yes, Python functions can return multiple values using tuples.
def get_coordinates(): return 10, 20 # Returning multiple values as a tuple x, y = get_coordinates() print(x, y) # Output: 10 20
What is a lambda function in Python?
A lambda
function is an anonymous (nameless) function that can have multiple arguments but only one expression. It is used for short, simple operations.
square = lambda x: x * x print(square(4)) # Output: 16
How do lambda functions differ from normal functions?
- Name: A lambda function is anonymous (has no name), while a normal function has a defined name.
- Syntax: A lambda function is written in a single line, while a normal function can span multiple lines.
- Use Case: Lambda functions are used for short, simple tasks, whereas normal functions handle complex logic.
- Return: In a lambda function, the return is implicit, whereas in a normal function, you must explicitly use the return keyword.
Normal Function:
def multiply(a, b): return a * b
Lambda Function:
multiply_lambda = lambda a, b: a * b
Can a lambda function return multiple values?
No, a lambda
function can only have one expression, but you can return multiple values using a tuple
.
multi_return = lambda x, y: (x + y, x - y) print(multi_return(10, 5)) # Output: (15, 5)
How can you use a lambda function with map(), filter(), or reduce()?
Lambda functions are commonly used with built-in functions like map()
, filter()
, and reduce()
.map()
(applies a function to each element in a list):
numbers = [1, 2, 3, 4] squared = list(map(lambda x: x * x, numbers)) print(squared) # Output: [1, 4, 9, 16]
filter()
(filters elements based on a condition):
numbers = [1, 2, 3, 4, 5, 6] even_numbers = list(filter(lambda x: x % 2 == 0, numbers)) print(even_numbers) # Output: [2, 4, 6]
reduce()
(reduces a list to a single value):
from functools import reduce numbers = [1, 2, 3, 4] product = reduce(lambda x, y: x * y, numbers) print(product) # Output: 24
What is a decorator in Python?
A decorator
is a function that takes another function and extends or alters its behaviour. It allows modification of a function or class method without changing the original code.
def decorator(func): def wrapper(): print("Before the function call") func() print("After the function call") return wrapper @decorator def say_hello(): print("Hello!") say_hello()
Output:
Before the function call Hello! After the function call
How do closures work in Python?
A closure
is a function that "remembers" its lexical scope even when the function is executed outside that scope. This is useful when we want to retain some data across function calls.
def outer_func(x): def inner_func(y): return x + y return inner_func closure = outer_func(10) print(closure(5)) # Output: 15
Can you explain the concept of a decorator with arguments?
A decorator
can also accept arguments to customize its behavior. This is done by adding another level of function nesting.
def repeat_decorator(n): def decorator(func): def wrapper(*args, **kwargs): for _ in range(n): func(*args, **kwargs) return wrapper return decorator @repeat_decorator(3) def greet(name): print(f"Hello, {name}!") greet("Alice")
Output:
Hello, Alice! Hello, Alice! Hello, Alice!
What is the difference between a decorator and a closure?
- A decorator is a function that modifies another function, often used for logging, access control, etc.
- A closure is a nested function that has access to variables from the outer function even after the outer function has finished execution.
How do you handle function arguments in a decorator?
You can pass function arguments to a decorator using the *args
and **kwargs
syntax to capture any number of arguments.
def log_args(func): def wrapper(*args, **kwargs): print(f"Arguments were: {args}, {kwargs}") return func(*args, **kwargs) return wrapper @log_args def add(a, b): return a + b add(2, 3)
Output:
Arguments were: (2, 3), {}
What are some practical uses of decorators in Python?
Decorators are commonly used for:
- Logging: Tracking function calls and inputs/outputs.
- Authentication: Checking if a user is authenticated before executing a function.
- Memoization: Caching the results of expensive function calls.
Memoization:
def memoize(func): cache = {} def wrapper(*args): if args not in cache: cache[args] = func(*args) return cache[args] return wrapper @memoize def slow_function(n): print("Calculating...") return n * n print(slow_function(4)) # Calculates and stores the result print(slow_function(4)) # Returns the cached result
Output:
Calculating... 16 16
What is a class in Python?
A class is a blueprint for creating objects. It defines attributes (variables) and methods (functions) that describe the behaviour of an object.
class Car: def __init__(self, brand, model): self.brand = brand self.model = model
What is an object in Python?
An object is an instance of a class that holds actual values for attributes.
my_car = Car("Toyota", "Camry") print(my_car.brand) # Output: Toyota
How do you create an object of a class?
An object is created by calling the class name followed by parentheses.
obj = Car("Ford", "Mustang") print(obj.model) # Output: Mustang
What is the difference between a class and an object?
A class defines a structure, while an object is an instance of that structure with real values.
class Dog: def __init__(self, name): self.name = name dog1 = Dog("Buddy") # Object dog2 = Dog("Charlie") # Object print(dog1.name) # Output: Buddy print(dog2.name) # Output: Charlie
What is self in a class?self
represents the instance of the class and allows access to attributes and methods.
class Person: def __init__(self, name): self.name = name def greet(self): return f"Hello, my name is {self.name}" p = Person("Alice") print(p.greet()) # Output: Hello, my name is Alice
How do you define a class attribute?
A class attribute is shared among all instances of the class.
class Example: count = 0 # Class attribute print(Example.count) # Output: 0
What happens if you don't use self in instance methods?
Python will not know which object’s attributes to access, leading to an error.
class Test: def set_value(value): # Missing self self.value = value # Error obj = Test() # obj.set_value(10) # TypeError: set_value() takes 1 positional argument but 2 were given
Can an object modify class attributes?
Yes, but it will create an instance-specific attribute instead of modifying the class attribute.
class Example: count = 0 # Class attribute obj1 = Example() obj1.count = 5 # Creates instance attribute, does not change class attribute print(Example.count) # Output: 0 print(obj1.count) # Output: 5
What is the purpose of the __init__ method?
The __init__
method initializes an object’s attributes when an instance is created.
class Student: def __init__(self, name, age): self.name = name self.age = age s1 = Student("John", 20) print(s1.name, s1.age) # Output: John 20
Can you create an object without a class?
No, an object must always be an instance of a class. However, you can use dynamic types like dictionaries to store object-like data.
obj = {"name": "John", "age": 25} print(obj["name"]) # Output: John
What is an attribute in Python?
An attribute is a variable that belongs to an object or a class.
class Car: def __init__(self, brand): self.brand = brand # Instance attribute car1 = Car("Toyota") print(car1.brand) # Output: Toyota
What are instance attributes?
Attributes specific to an instance, defined using self inside __init__
.
class Dog: def __init__(self, name): self.name = name dog1 = Dog("Max") dog2 = Dog("Bella") print(dog1.name) # Output: Max print(dog2.name) # Output: Bella
What are class attributes?
Attributes shared across all instances of a class.
class Example: count = 0 # Class attribute print(Example.count) # Output: 0
What is a method in Python?
A function inside a class that operates on the instance or class.
class Math: def add(self, a, b): return a + b obj = Math() print(obj.add(2, 3)) # Output: 5
What is an instance method?
A method that operates on an instance and uses self
.
class Person: def greet(self): return "Hello" p = Person() print(p.greet()) # Output: Hello
What is a class method?
A method that operates on the class, defined using @classmethod
and cls
.
class Example: value = 0 @classmethod def change(cls, new_value): cls.value = new_value Example.change(10) print(Example.value) # Output: 10
What is a static method?
A method that doesn’t depend on the instance or class, defined using @staticmethod
.
class Math: @staticmethod def add(a, b): return a + b print(Math.add(3, 4)) # Output: 7
What is the difference between instance, class, and static methods?
- Instance methods: Modify instance attributes (self).
- Class methods: Modify class attributes (cls).
- Static methods: Independent utility functions.
class Example: count = 0 def instance_method(self): return "Instance Method" @classmethod def class_method(cls): return "Class Method" @staticmethod def static_method(): return "Static Method" obj = Example() print(obj.instance_method()) # Output: Instance Method print(Example.class_method()) # Output: Class Method print(Example.static_method()) # Output: Static Method
Can methods have default parameters?
Yes, methods can have default parameters like normal functions.
class Example: def greet(self, name="Guest"): return f"Hello, {name}" obj = Example() print(obj.greet()) # Output: Hello, Guest print(obj.greet("Alice")) # Output: Hello, Alice
How can you call a method without creating an instance?
By using a class method or static method.
class Example: @staticmethod def show(): return "Static method" print(Example.show()) # Output: Static method
What is inheritance in Python?
Inheritance allows a class to derive attributes and methods from another class, promoting code reusability.
class Animal: def sound(self): return "Some sound" class Dog(Animal): # Dog inherits from Animal def sound(self): return "Bark" d = Dog() print(d.sound()) # Output: Bark
What are the different types of inheritance in Python?
Python supports single, multiple, multilevel, hierarchical, and hybrid inheritance.
class A: def method_A(self): return "Method A" class B(A): # Single Inheritance def method_B(self): return "Method B" obj = B() print(obj.method_A()) # Output: Method A print(obj.method_B()) # Output: Method B
What is the difference between parent class and child class?
- Parent Class (Super Class): The class being inherited from.
- Child Class (Sub Class): The class that inherits from another class.
class Parent: def display(self): return "Parent Class" class Child(Parent): def show(self): return "Child Class" obj = Child() print(obj.display()) # Output: Parent Class print(obj.show()) # Output: Child Class
What is method overriding in Python?
Method overriding occurs when a child class provides a specific implementation of a method already defined in the parent class.
class Vehicle: def move(self): return "Vehicles can move" class Car(Vehicle): def move(self): return "Cars can drive" obj = Car() print(obj.move()) # Output: Cars can drive
What is multiple inheritance?
A class inheriting from more than one class.
class A: def method_A(self): return "Class A" class B: def method_B(self): return "Class B" class C(A, B): # Multiple Inheritance pass obj = C() print(obj.method_A()) # Output: Class A print(obj.method_B()) # Output: Class B
What is polymorphism in Python?
Polymorphism allows different classes to have the same method name but different implementations.
class Bird: def sound(self): return "Chirp" class Dog: def sound(self): return "Bark" def make_sound(animal): print(animal.sound()) make_sound(Bird()) # Output: Chirp make_sound(Dog()) # Output: Bark
What is method overloading in Python?
Python does not support traditional method overloading, but it can be achieved using default arguments.
class Example: def display(self, a=None, b=None): if a and b: return a + b elif a: return a else: return "No arguments" obj = Example() print(obj.display(5, 10)) # Output: 15 print(obj.display(5)) # Output: 5 print(obj.display()) # Output: No arguments
What is the super() function?super()
is used to call the parent class’s method inside a child class.
class Parent: def show(self): return "Parent method" class Child(Parent): def show(self): return super().show() + " overridden by Child" obj = Child() print(obj.show()) # Output: Parent method overridden by Child
What is an abstract class?
An abstract class has at least one abstract method (a method with no implementation) and cannot be instantiated.
from abc import ABC, abstractmethod class Animal(ABC): @abstractmethod def sound(self): pass class Dog(Animal): def sound(self): return "Bark" d = Dog() print(d.sound()) # Output: Bark
What is an interface in Python?
Python doesn't have built-in interfaces like other languages (e.g., Java). However, abstract classes can be used to simulate interface behaviour by defining methods without implementations, forcing subclasses to implement them.
from abc import ABC, abstractmethod class Shape(ABC): @abstractmethod def area(self): pass # Abstract method, no implementation class Circle(Shape): def area(self): return "Circle area" c = Circle() print(c.area()) # Output: Circle area # Here, Shape acts like an interface, defining a method area() that must be implemented by any subclass.
What is encapsulation in Python?
Encapsulation restricts direct access to class attributes and methods to protect data integrity.
class Example: def __init__(self): self.__secret = "Hidden" def reveal(self): return self.__secret obj = Example() print(obj.reveal()) # Output: Hidden
How do you make an attribute private in Python?
You can make an attribute private by prefixing it with double underscores (__
). This prevents direct access from outside the class, ensuring data protection.
class Account: def __init__(self, balance): self.__balance = balance # Private attribute def get_balance(self): return self.__balance # Accessed via method acc = Account(1000) print(acc.get_balance()) # Output: 1000 # Here, __balance is private, so it cannot be accessed directly using acc.__balance. Instead, we use the get_balance() method to retrieve its value.
What is abstraction in Python?
Abstraction hides implementation details and only shows essential features.
from abc import ABC, abstractmethod class Vehicle(ABC): @abstractmethod def move(self): pass class Car(Vehicle): def move(self): return "Car is moving" obj = Car() print(obj.move()) # Output: Car is moving
What is the difference between encapsulation and abstraction?
- Encapsulation: Restricts access to internal details.
- Abstraction: Hides complex implementation and shows only the necessary parts.
Can private attributes be accessed outside the class?
No, but they can be accessed using name mangling (_ClassName__
attribute).
class Example: def __init__(self): self.__private = "Secret" obj = Example() print(obj._Example__private) # Output: Secret
What is the __init__ method?
It initializes object attributes when an instance is created.
class Person: def __init__(self, name): self.name = name p = Person("Alice") print(p.name) # Output: Alice
What is the __str__ method?
It returns a string representation of an object.
class Example: def __str__(self): return "This is an Example object" obj = Example() print(obj) # Output: This is an Example object
What is the __repr__ method?
It provides a developer-friendly string representation of an object.
class Example: def __repr__(self): return "Example()" obj = Example() print(repr(obj)) # Output: Example()
What is operator overloading?
Operator overloading allows defining custom behaviour for operators.
class Number: def __init__(self, value): self.value = value def __add__(self, other): return self.value + other.value n1 = Number(5) n2 = Number(10) print(n1 + n2) # Output: 15