ReactJS
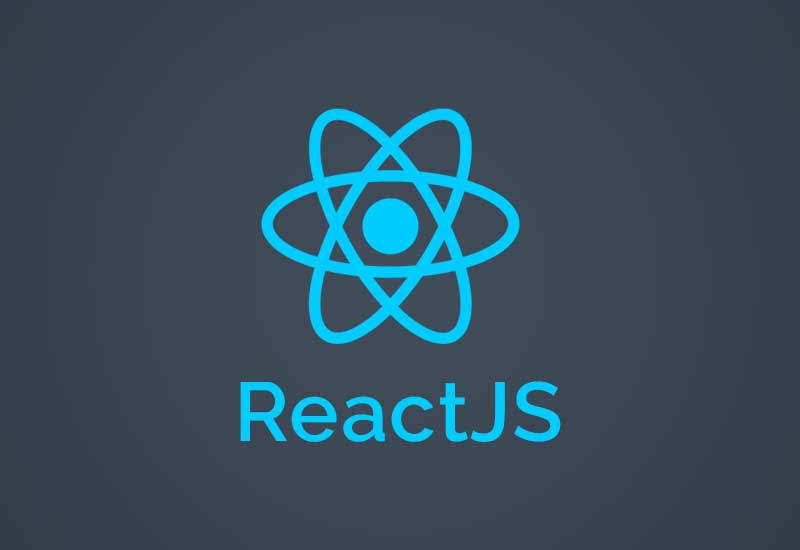
ReactJS Training Course Content
JavaScript Fundamentals
- New Features of ES6 & ES7
- Understanding let & const
- Using arrow functions
- Default arguments
- Rest Parameters
- Spread operator
- Getters and setters
- Understanding promises
- Map, filter, reduce methods in Javascriptt
ReactJS Fundamentals-1
- Introduction to ReactJS
- Understanding DOM
- Virtual DOM Vs Real DOM
- Setting Up React Environment
- Understanding NPM
- NPM Commands for React
- Understanding React Elements
ReactJS Fundamentals-2
- Introduction to Components
- Understanding Function Components
- Understanding Class Components
- Props in React
- Understanding State in React
- Iterating through Lists
- Handling Events
- Working with REST API
Components Deep dive
- Understanding Component Based App Development
- Components Interaction
- Parent Components, Child Components
- Component Communication
- Smart Components Vs Dumb Components
- Pure Components
- Impure Components
- Component Lifecycle Methods
- Higher Order Components
- Controlled Components Vs UnControlled Components
ReactJS Hooks
- Understanding Hooks in React
- use State
- use Effect
- use Context
- Custom Hooks
- Custom Hooks from NPM
ReactJS Concepts
- Building Forms in React
- Form Validation in React
- Lifting State Up
- Error Boundaries
- Portals
- Profiler
- Refs
- Render Props
- Master Pages
- Reconciliation
- Routing
- Protected Routes
- Prevent Transition
- Conditional Rendering
- Composition Vs Inheritance
- State Management
- Implementing Redux in app
- Creating store and using in app
- Defining reducers and actions
- Connecting components to store
- Unit Testing the React App
- Implementing Authentication
- Integration of 3rd Party Modules
- Building and Deploying React App